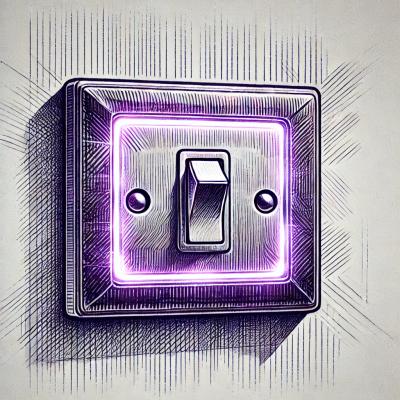
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
ember-cli-typescript
Advanced tools
Adds precompilation of TypeScript files and all the basic generation types to the `ember generate` command.
ember-cli-typescript is an addon for Ember.js that enables TypeScript support in Ember applications. It provides a seamless integration of TypeScript into the Ember build pipeline, allowing developers to write their Ember code in TypeScript, which offers static type checking and other benefits.
TypeScript Integration
This feature allows you to write Ember components using TypeScript. The code sample demonstrates a simple Glimmer component written in TypeScript, with type annotations for the component's arguments.
import Component from '@glimmer/component';
interface MyComponentArgs {
name: string;
}
export default class MyComponent extends Component<MyComponentArgs> {
get greeting(): string {
return `Hello, ${this.args.name}!`;
}
}
Type Checking
Type checking ensures that the types of the arguments passed to components are correct. The code sample shows a type error when an incorrect type is passed to the component.
import Component from '@glimmer/component';
interface MyComponentArgs {
name: string;
}
export default class MyComponent extends Component<MyComponentArgs> {
get greeting(): string {
return `Hello, ${this.args.name}!`;
}
}
// This will cause a type error
let component = new MyComponent({ name: 123 });
TypeScript Configuration
This feature allows you to configure TypeScript settings for your Ember project. The code sample shows a typical tsconfig.json file used to configure the TypeScript compiler options and include paths.
{
"compilerOptions": {
"target": "es6",
"module": "esnext",
"strict": true,
"noImplicitAny": true,
"moduleResolution": "node",
"baseUrl": ".",
"paths": {
"*": ["types/*"]
}
},
"include": [
"app/**/*",
"tests/**/*"
]
}
ember-cli-coffeescript is an addon that allows you to write your Ember.js code using CoffeeScript. While it provides a similar integration for a different language, it does not offer the same level of static type checking and tooling support as TypeScript.
ember-cli-babel is an addon that integrates Babel into the Ember build pipeline, allowing you to use the latest JavaScript features in your Ember code. While it does not provide type checking, it is a popular choice for modern JavaScript development in Ember.
ember-cli-flowtype is an addon that integrates Flow, a static type checker for JavaScript, into Ember projects. It offers similar type checking capabilities as TypeScript but is less commonly used in the Ember ecosystem.
Adds precompilation of TypeScript files and all the basic generation
types to the ember generate
command.
It was inspired (and forked from) the CoffeeScript addon (https://github.com/kimroen/ember-cli-coffeescript).
Note: This is under development and probably doesn't work as expected. PRs welcome!
ember generate
ember install:addon ember-cli-typescript
NB: This addon requires ember-cli version 0.2.0-beta.1
and up.
FAQs
Allow Ember apps to use TypeScript files.
The npm package ember-cli-typescript receives a total of 315,264 weekly downloads. As such, ember-cli-typescript popularity was classified as popular.
We found that ember-cli-typescript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.