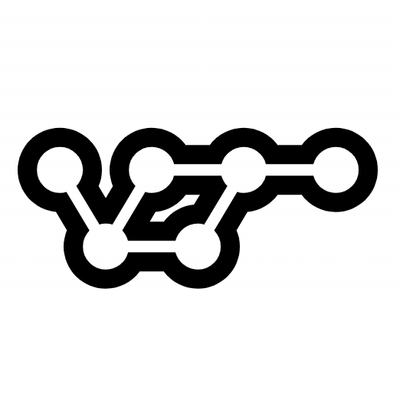
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
🚀 Simple HTML templating for expressjs.
npm install expandify
First create a HTML file that will serve as your template (index.html).
<!DOCTYPE html>
<html>
<body>
<h1>Hey expandify templates!</h1>
</body>
</html>
Then create a express application with expandify (index.js).
npm i express
const {renderFile} = require("expandify");
const express = require("express");
const path = require("path");
const app = express();
app.get("/", (req, res) => {
res.send(renderFile(path.join(__dirname, "index.html")));
});
app.listen(8080);
The renderFile
function takes the path to your template, and returns a string as your compiled template. You can use the res.send()
function to display the compiled template's HTML. You can also use a string instead of another file for your template with the render
function. However, these docs will use the renderFile
function for consistency. It is also a good idea to seperate your templates into another file.
Once you run node index.js
, head to localhost:8080
and you will see Hey expandify templates!
on the screen!
You can embed any values or expressions into your templates:
<!DOCTYPE html>
<html>
<body>
<h1>1+1 is equal to {{1+1}}</h1>
</body>
</html>
You can also embed variables from your javascript code:
<!DOCTYPE html>
<html>
<body>
<h1>Hey {{name}}</h1>
<p>{{greeting}}</p>
</body>
</html>
Then, when you call renderFile()
pass the variables like this:
renderFile(__dirname + "/index.html", { name: "Bob", greeting: "How's life!" });
You can embed lists (which will be joined), or map through them. This must stay on one line:
<!DOCTYPE html>
<html>
<body>
<ul>
{todos.map(todo => `<li>${todo}</li>`)}
</ul>
</body>
</html>
renderFile(__dirname + "/index.html", { todos: ["Do chores", "Do homework"] });
Or embed JSON, which will be stringified for you:
<!DOCTYPE html>
<html>
<body>
<h1>My cool JSON</h1>
{{{key: "value"}}}
</body>
</html>
You can set an attribute equal to any expression with the $:attribute
directive:
<!DOCTYPE html>
<html>
<body>
<input type="number" $:value="1+1" />
</body>
</html>
Or set an attribute equal to a variable passed in through your javascript code:
<!DOCTYPE html>
<html>
<body>
<input type="text" $:placeholder="myPlaceholder" />
</body>
</html>
renderFile(__dirname + "/index.html", { myPlaceholder: "Hey!" });
A shortcut for assigning a variable to a attribute with the same name is $:attribute:
:
<!DOCTYPE html>
<html>
<body>
<input type="text" $:placeholder: />
</body>
</html>
renderFile(__dirname + "/index.html", { placeholder: "Hey!" });
To write embedded styles with scss, use the <style lang="scss">
tag:
<!DOCTYPE html>
<html>
<head>
<style lang="scss">
$size: 10rem;
body {
font-size: $size;
}
</style>
</head>
<body>
Large text!
</body>
</html>
You dont have to do anything else, expandify will automatically compile the SCSS for you!
expandify is MIT-licensed open-source software created by Bharadwaj Duggaraju.
FAQs
🚀 Simple HTML templating for expressjs.
The npm package expandify receives a total of 0 weekly downloads. As such, expandify popularity was classified as not popular.
We found that expandify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.