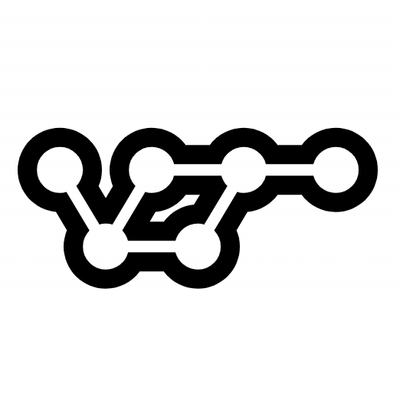
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
fidel-react-native
Advanced tools
This SDK is a bridge between React Native and Fidel's native iOS and Android SDKs. It helps you to add card linking technology to your React Native apps in minutes. It captures credit/debit card numbers securely and links them to your programs.
$ npm install fidel-react-native --save
Libraries
➜ Add Files to [your project's name]
node_modules
➜ fidel-react-native
and add Fidel.xcodeproj
libFidel.a
to your project's Build Phases
➜ Link Binary With Libraries
Build Settings
, make sure to set YES
for Always Embed Swift Standard Libraries
. That's because, by default, your project might not need it. It needs to be YES
because otherwise the project will not find the Swift libraries to be able to run our native iOS SDK.You can use Cocoapods or install the library as a dynamic library.
Podfile
in your ios/
folder of your React Native project. It should include the following dependency:use_frameworks!
pod 'Fidel'
Check our example Podfile to see the simplest Podfile
you need to link with our native iOS SDK.
If you use an older Swift version, please check our iOS SDK README. You'll find a suitable version you should set for your Cocoapods dependency.
Podfile
, make sure to set the iOS platform 9.1 or above:# Use iOS 9.1 and above
platform :ios, '9.1'
pod install
in your terminal..xcworkspace
created by Cocoapods when you run your iOS app. React Native should use it by default.android/settings.gradle
:include ':fidel-react-native'
project(':fidel-react-native').projectDir = new File(rootProject.projectDir, '../node_modules/fidel-react-native/android')
android/app/build.gradle
:implementation project(':fidel-react-native')
android/app/src/main/java/[...]/MainApplication.java
import com.reactlibrary.FidelPackage;
to the imports at the top of the filenew FidelPackage()
to the list returned by the getPackages()
method:protected List <ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new FidelPackage(),
//you might have other Packages here as well.
);
}
android/build.gradle
:allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
minSdkVersion
is the same or higher than the minSdkVersion
of our native Android SDK:buildscript {
ext {
...
minSdkVersion = 19
...
}
}
For a physical device you need to search on Google for Google Play Services. There will be a link that takes you to the Play Store and from there you will see a button to update it (do not search within the Play Store).
Import Fidel in your RN project:
import Fidel from 'fidel-react-native';
Set up Fidel with your API key and your program ID. Without them you can't open the Fidel card linking UI:
Fidel.setup({
apiKey:'your api key',
programId: 'your program id'
})
Set the options that create the experience of linking a card with Fidel:
const myImage = require('./images/fdl_test_banner.png');
const resolveAssetSource = require('react-native/Libraries/Image/resolveAssetSource');
const resolvedImage = resolveAssetSource(myImage);
//this is the default value for supported card schemes,
//but you can remove the support for some of the card schemes if you want to
const cardSchemes = new Set([
Fidel.CardScheme.visa,
Fidel.CardScheme.mastercard,
Fidel.CardScheme.americanExpress
]);
Fidel.setOptions ({
bannerImage: resolvedImage,
country: Fidel.Country.sweden,
supportedCardSchemes: Array.from(cardSchemes),
autoScan: false,
metaData: {'meta-data-1': 'value1'}, //additional data to pass with the card
companyName: 'My RN Company', //the company name displayed in our
deleteInstructions: 'My custom delete instructions!',
privacyUrl: 'https://fidel.uk',
});
Open the card linking view by calling:
Fidel.openForm((error, result) => {
if (error) {
console.error(error);
} else {
console.info(result);
}
});
Both result
and error
are objects that look like in the following examples:
{
accountId: "the-account-id"
countryCode: "GBR" // the country selected by the user, in the Fidel SDK form
created: "2019-04-22T05:26:45.611Z"
expDate: "2023-12-31T23:59:59.999Z" // the card expiration date
expMonth: 12 // for your convenience, this is the card expiration month
expYear: 2023 // for your convenience, this is the card expiration year
id: "card-id" // the card ID as registered on the Fidel platform
lastNumbers: "4001" //last numbers of the card
live: false
mapped: false
metaData: {meta-data-1: "value1"} //the meta data that you specified for the Fidel SDK
programId: "your program ID, as specified for the Fidel SDK"
scheme: "visa"
type: "visa"
updated: "2019-04-22T05:26:45.611Z"
}
{
code: "item-save" // the code of the error
date: "2019-04-22T05:34:04.621Z" // the date of the card link request
message: "Item already exists" // the message of the error
}
Use this option to customize the topmost banner image with the Fidel UI. Your custom asset needs to be resolved in order to be passed to our native module:
const myImage = require('./images/fdl_test_banner.png');
const resolveAssetSource = require('react-native/Libraries/Image/resolveAssetSource');
const resolvedImage = resolveAssetSource(myImage);
Fidel.setOptions ({
bannerImage: resolvedImage
}
Set a default country the SDK should use with:
Fidel.setOptions ({
country: Fidel.Country.unitedKingdom
});
When you set a default country, the card linking screen will not show the country picker UI. The other options, for now, are: .unitedStates
, .ireland
, .sweden
, .japan
, .canada
.
We currently support Visa, Mastercard and AmericanExpress, but you can choose to support only one, two or all three. By default the SDK is configured to support all three.
If you set this option to an empty array or to null
, of course, you will not be able to open the Fidel UI. You must support at least one our supported card schemes.
Please check the example below:
//this is the default value for supported card schemes,
//but you can remove the support for some of the card schemes if you want to
const cardSchemes = new Set([
Fidel.CardScheme.visa,
Fidel.CardScheme.mastercard,
Fidel.CardScheme.americanExpress
]);
Fidel.setOptions ({
supportedCardSchemes: Array.from(cardSchemes)
});
Set this property to true
, if you want to open the card scanning UI immediately after executing Fidel.openForm
. The default value is false
.
Fidel.setOptions ({
autoScan: true
});
Use this option to pass any other data with the card data:
Fidel.setOptions ({
metaData: {'meta-data-key-1': 'value1'}
});
Set your company name as it will appear in our consent checkbox text. Please set it to a maximum of 60 characters.
Fidel.setOptions ({
companyName: 'Your Company Name'
});
Write your custom opt-out instructions for your users. They will be displayed in the consent checkbox text as well.
Fidel.setOptions ({
deleteInstructions: 'Your custom card delete instructions!'
});
This is the privacy policy URL that you can set for the consent checkbox text.
Fidel.setOptions ({
privacyUrl: 'https://fidel.uk',
});
In the test environment please use our VISA, Mastercard or American Express test card numbers. You must use a test API Key for them to work.
VISA: 4444000000004*** (the last 3 numbers can be anything)
Mastercard: 5555000000005*** (the last 3 numbers can be anything)
American Express: 3400000000003** or 3700000000003** (the last 2 numbers can be anything)
The FIDEL SDK is in active development, we welcome your feedback!
Get in touch:
GitHub Issues - For SDK issues and feedback FIDEL Developers Slack Channel - https://fidel-developers-slack-invites.herokuapp.com - for personal support at any phase of integration
FAQs
Fidel's React Native bridge library for iOS and Android.
We found that fidel-react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.