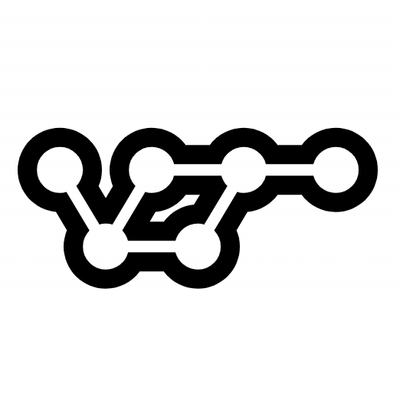
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
firex-store
Advanced tools
If you use this npm, you can reference firestore data, easily
It is inspired by vuexfire
node v8.9.4 ~
npm install --save firex-store
others comming soon
Return values or state values bounded to Firestore has docId
(documentId in Firestore) property.
A store module cannot subscribe to more than one 'collection' and 'document'
If you want to subscribe again after unsubscribing 'collection', set the property of the store you want to subscribe to []
and then subscribe.
.....
firebase.initializeApp({
apiKey: [your firebase api key],
projectId: [your project id],
.....
})
export const firestore = firebase.firestore()
firestoreMutations
Ex. Subscribe collection
export default {
namespaced: true,
state: {
comments: [],
comment: null
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' })
},
.....
}
method: firestoreSubscribeAction
or firestoreSubscribeActions
firestoreSubscribeActions
is deprecated. It will be removed at ^1.0.0~
argments:
Ex. Subscribe collection
// modules: comment
export default {
namespaced: true,
state: {
comments: [],
comment: null
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' })
},
actions: {
...firestoreSubscribeAction({ ref: firestore.collection('/comments') })
}
.....
}
Ex. Subscribe collection
<script>
import { actionTypes } from 'firex-store'
export default {
name: 'Comments',
created() {
this.$store.dispatch(`comment/${actionTypes.collection.SUBSCRIBE}`)
}
}
</script>
firestoreMutations
Ex. Subscribe collection
export default {
namespaced: true,
state: {
comments: [],
comment: null
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' })
},
.....
}
subscribeFirestore
in custom-actionssubscribeFirestore
export default {
namespaced: true,
state: {
comments: [],
comment: null
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' })
},
actions: {
subscribe: ({ state, commit }) => {
subscribeFirestore({
state,
commit,
ref: firestore.collection('/comments'),
options
})
}
}
.....
}
Ex. Unsubscribe collection
firestoreUnsubscribeAction
in actionsmethod: firestoreUnsubscribeAction
or firestoreUnsubscribeActions
firestoreUnsubscribeActions
is deprecated. It will be removed at ^1.0.0~
argments:
export default {
namespaced: true,
state: {
comments: [],
comment: null
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' })
},
actions: {
...firestoreSubscribeAction({ ref: firestore.collection('/comments') }),
...firestoreUnsubscribeAction({ type: 'collection' })
}
.....
}
<script>
import { actionTypes } from 'firex-store'
export default {
name: 'Comments',
created() {
this.$store.dispatch(`comment/${actionTypes.collection.UNSUBSCRIBE}`)
}
}
</script>
method: unsubscribeFirestore
argments:
export default {
namespaced: true,
state: {
comments: [],
comment: null
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' })
},
actions: {
subscribe: ({ state, commit }) => {
subscribeFirestore({
state,
commit,
ref: firestore.collection('/comments'),
options
})
},
unsubscribe: ({ state }) => {
unsubscribeFirestore({
state,
type: 'collection'
})
}
}
.....
}
method: findFirestore
argments:
Return null
if data not found
EX. Call in Store Action, to fetch collection
export default {
namespaced: true,
state: {},
getters: {},
mutations: {},
actions: {
fetchComments: async ({ commit }) => {
const ref = firestore.collection('/comments')
const result = await findFirestore({ ref })
commit(***, result)
}
}
}
Options
mapper:
errorHandler
console.error(error)
CompletionHandler
OnCompleted ※ It is deprecated and removed on v1.0.0~
afterMutationCalled
subscribeFirestore
and subscribeFirestoreActions
only.notFoundHandler
Ex.
const mapUser = (data) => ({
id: data.id
name: data.name
.....
})
const errorHandler = (error) => {
console.error(`[App Name]: ${error}`)
}
const completionHandler = () => {
console.log('completed!')
}
const afterMutationCalled = (payload) => {
/**
* payload = {
* data: { docId: string | null, [key: string]: any },
* isLast: boolean,
* [key: string]: any
* }
* */
if (payload.isLast === false) {
commit('SET_LOADING', true)
} else if (payload.isLast === true) {
commit('SET_LOADING', false)
}
}
const notFoundHandler = (type, isAll) => {
console.log('not found')
}
const notFoundHandler = (type, isAll) => {
console.log('not found')
}
export default {
namespaced: true,
state: {
comments: [],
comment: null,
isLoading: false
},
mutations: {
...firestoreMutations({ statePropName: 'comments', type: 'collection' }),
SET_LOADING(state, isLoading) {
state.isLoading = isLoading
}
},
actions: {
subscribe: ({ state, commit }) => {
subscribeFirestore({
state,
commit,
ref: firestore.collection('/comments'),
options: {
mapper: mapUser,
errorHandler,
completionHandler,
// onCompleted, <- deprecated
afterMutationCalled,
notFoundHandler
}
})
}
}
.....
}
FAQs
Subscribe and write firestore data, easily
The npm package firex-store receives a total of 2 weekly downloads. As such, firex-store popularity was classified as not popular.
We found that firex-store demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.