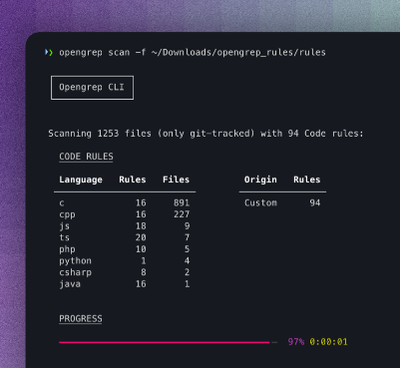
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
google-slides
Advanced tools
npm install google-slides
import { API, Presentation } from 'google-slides'
const api = new API('path/to/credentials.json')
const presentationId = '1yMEqtOta984dwNyJoeU92tsC5x7GV2fQK7V4wJc60Mg'
const template = new Presentation({ id: presentationId }, api)
template.copy()
.then(newPresentation => console.log(`New presentation created with name ${newPresentation.name} and ID ${newPresentation.id}.`))
.catch(e => console.log('Copy error:', e))
See API.sharePresentation.
Instead of api.sharePresentation
use presentation.share
and don't pass id
.
See API.presentationBatchUpdate.
Instead of api
use presentation
and don't pass presentationId
.
import { API } from 'google-slides'
const api = new API('path/to/credentials.json')
api.copyPresentation(id)
.then(newPresentation => console.log(`name: ${newPresentation.name}, id: ${newPresentation.id}`))
const emailAddress = 'wpbdry@gmail.com'
const role = 'writer' // One of: owner | organizer | fileOrganizer | writer | commenter | reader
const type = 'user' // One of: user | group | domain | anyone
const sendNotificationEmails = false
api.sharePresentation(id, emailAddress, role, type, sendNotificationEmails)
.then(() => console.log(`Presentation with ID ${id} successfully shared with ${emailAddress}!`))
import { API, TextReplacement, ShapeReplacementWithImage } from 'google-slides'
const api = new API('path/to/credentials.json')
api.presentationBatchUpdate(presentationId, [
new TextReplacement('{{client-name}}', 'My Client'),
new ShapeReplacementWithImage('{{client-logo}}', logoUrl)
])
.catch(error => doSomething(error))
Replaces all instances of text matching a criterion with replaceText
. \
const text = '{{some-text-to-replace}}'
const replaceText = 'Replacement Text'
const matchCase = true // default
const textReplacement = new TextReplacement(text, replaceText, matchCase)
Replaces all shapes that match the given criteria with the provided image.
const text = '{{text-contained-within-shape}}'
const imageUrl = 'https://example.com/my-img'
const matchCase = true // default
const replaceMethod = 'CENTER_INSIDE' // default
const shapeReplacement = new ShapeReplacementWithImage(text, imageUrl, matchCase, replaceMethod)
The API class implements underlying services, which can be accessed directly if they are await
ed.
This provides access to further functions from the googleapis
module, which may not be implemented here.
import { API } from 'google-slides'
(async function() {
const api = new API('path/to/credentials.json')
(await api.driveService).doSomething()
})()
The driveService
object here is equivelant to the drive
object used in all the Node.js snippets in the
Google Drive API V3 documentation
import { API } from 'google-slides'
(async function() {
const api = new API('path/to/credentials.json')
(await api.slidesService).doSomething()
})()
The slidesService
object here is equivelant to the slides
or this.slidesService
objects used in all the Node.js snippets in the
Google Slides API documentation
await
?When new API('path/to/credentials.json')
is called, the API logs in with the provided credentials, and initialized the underlying services.
Since logging in happens asynchronously, the services may not yet exist by the time they are used. These services are await
ed every time
they are used in the built in methods. You only need to await
each service the first time you use it in any single promise chain.
FAQs
NPM package for some simple Google Slides operations
The npm package google-slides receives a total of 5 weekly downloads. As such, google-slides popularity was classified as not popular.
We found that google-slides demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.