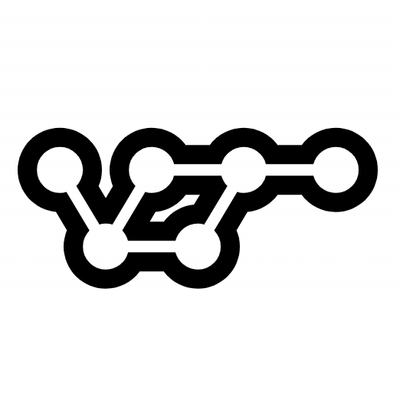
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
graphql-rate-limit
Advanced tools
A GraphQL directive to add basic but granular rate limiting to your Queries or Mutations.
max
per window
argumentsyarn add graphql-rate-limit
directive @rateLimit(
max: Int,
window: String,
message: String,
identityArgs: [String],
) on FIELD_DEFINITION
type Query {
# Rate limit to 5 per second
getItems: [Item] @rateLimit(window: "1s", max: 5)
# Rate limit access per item ID
getItem(id: ID!): Item @rateLimit(identityArgs: ["id"])
}
type Mutation {
# Rate limit with a custom error message
createItem(title: String!): Item @rateLimit(message: "You are doing that too often.")
# Rate limit access per item.id
updateItem(item: Item!): Item @rateLimit(identityArgs: ["item.id"])
}
Create a configured GraphQLRateLimit class.
const { createRateLimitDirective } = require('graphql-rate-limit');
// OR
import { createRateLimitDirective } from 'graphql-rate-limit';
const GraphQLRateLimit = createRateLimitDirective({
/**
* `identifyContext` is required and used to identify the user/client. The most likely cases
* are either using the context's request.ip, or the user ID on the context.
* A function that accepts the context and returns a string that is used to identify the user.
*/
identifyContext: (ctx) => ctx.user.id, // Or could be something like: return ctx.req.ip;
/**
* `store` is optional as it defaults to an InMemoryStore. See the implementation of InMemoryStore if
* you'd like to implement your own with your own database.
*/
store: new MyCustomStore(),
/**
* Generate a custom error message. Note that the `message` passed in to the directive will be used
* if its set.
*/
formatError: ({ fieldName }) => {
return `Woah there, you are doing way too much ${fieldName}`
}
});
Add GraphQLRateLimit to your GraphQL server configuration. Example using Apollo Server:
const server = new ApolloServer({
typeDefs,
resolvers,
schemaDirectives: {
rateLimit: GraphQLRateLimit
}
});
Note: If you are calling makeExecutableSchema
directly and passing in the schema
key to ApolloServer or similar, you should do the following:
const schema = makeExecutableSchema({
typeDefs,
resolvers
schemaDirectives: {
rateLimit: GraphQLRateLimit
}
});
const graphql = new ApolloServer({ schema });
Use in your GraphQL Schema.
# This must be added to the top of your schema.
directive @rateLimit(
max: Int,
window: String,
message: String,
identityArgs: [String],
) on FIELD_DEFINITION
type Query {
# Limit queries to getThings to 10 per minute.
getThings: [Thing] @rateLimit(max: 10, window: "6s")
}
type Query {
# Limit attempts to login with a particular email to 10 per 2 hours.
login(email: String!, password: String!): String @rateLimit(max: 10, window: "2h", identityArgs: ["email"])
}
window
Specify a time interval window that the max
number of requests can access the field. We use Zeit's ms
to parse the window
arg, docs here.
max
Define the max number of calls to the given field per window
.
identityArgs
If you wanted to limit the requests to a field per id, per user, use identityArgs
to define how the request should be identified. For example you'd provide just ["id"]
if you wanted to rate limit the access to a field by id
. We use Lodash's get
to access nested identity args, docs here.
message
A custom message per field. Note you can also use formatError
to customise the default error message if you don't want to define a single message per rate limited field.
It is recommended to use a persistent store rather than the default InMemoryStore. GraphQLRateLimit currently supports Redis as an alternative. You'll need to install Redis in your project first.
import { createRateLimitDirective, RedisStore } from 'graphql-rate-limit';
const GraphQLRateLimit = createRateLimitDirective({
identifyContext: ctx => ctx.user.id,
/**
* Import the class from graphql-rate-limit and pass in an instance of redis client to the constructor
*/
store: new RedisStore(redis.createClient())
});
FAQs
Add Rate Limiting To Your GraphQL Resolvers πββ
The npm package graphql-rate-limit receives a total of 27,773 weekly downloads. As such, graphql-rate-limit popularity was classified as popular.
We found that graphql-rate-limit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezerβs API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.