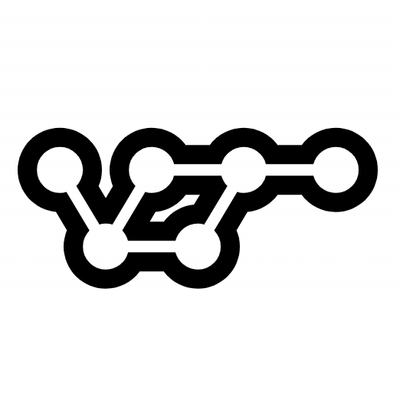
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
gulp-embed-json
Advanced tools
Gulp plugin to inline/embed JSON data into HTML files.
npm i --save-dev gulp-embed-json
const embedJSON = require('gulp-embed-json');
gulp.task('embedJSON', () =>
gulp.src('*.html')
.pipe(embedJSON())
.pipe(gulp.dest('dist/')));
This gulp task will inline/embed any scripts with JSON source attribute and respective mime type.
string | Array<string>
Provide custom mime types to specify which <script>
tags should be embedded.
application/json, application/ld+json
type="application/ld+json"
HTML layout
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json" src="data.json"></script>
<script type="application/ld+json" src="structured-data.json"></script>
<!-- ... -->
</body>
</html>
structured-data.json
{
"@context": "http://schema.org",
"@type": "SoftwareApplication",
"name": "gulp-embed-json"
}
Gulp task
const embedJSON = requrie('gulp-embed-json');
// ...
gulp.task('embedJSON', () =>
gulp.src('*.html')
.pipe(embedJSON({
mimeTypes: 'application/ld+json'
}))
.pipe(gulp.dest('dist/')));
Output
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json" src="data.json"></script>
<script type="application/ld+json">{"@context":"http://schema.org","@type":"SoftwareApplication","name":"gulp-embed-json"}</script>
<!-- ... -->
</body>
</html>
string
Provide the directory root where JSON files are located.
__dirname
The folder in which the module is executed.
HTML layout
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json" src="data.json"></script>
<!-- ... -->
</body>
</html>
Folder structure
/src
index.html
gulpfile.js
/assets
/json
data.json
Gulp task
const embedJSON = requrie('gulp-embed-json');
// ...
gulp.task('embedJSON', () =>
gulp.src('*.html')
.pipe(embedJSON({
root: './assets/json'
}))
.pipe(gulp.dest('dist/')));
boolean
Indicate whether or not to minify JSON data.
true
HTML layout
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json" src="data.json"></script>
<!-- ... -->
</body>
</html>
data.json
{
"foo": "bar"
}
Gulp task
const embedJSON = requrie('gulp-embed-json');
// ...
gulp.task('embedJSON', () =>
gulp.src('*.html')
.pipe(embedJSON({
minify: true // default
}))
.pipe(gulp.dest('dist/')));
Output
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json">{"foo":"bar"}</script>
<!-- ... -->
</body>
</html>
HTML layout
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json" src="data.json"></script>
<!-- ... -->
</body>
</html>
data.json
{
"foo": "bar"
}
Gulp task
const embedJSON = requrie('gulp-embed-json');
// ...
gulp.task('embedJSON', () =>
gulp.src('*.html')
.pipe(embedJSON({
minify: false
}))
.pipe(gulp.dest('dist/')));
Output
<html>
<head><!-- ... --></head>
<body>
<!-- ... -->
<script type="application/json">{
"foo": "bar"
}</script>
<!-- ... -->
</body>
</html>
string
Provide the encoding of your JSON files.
utf8
1.5.0
package.json
.FAQs
Gulp plugin to inline/embed JSON data into HTML files.
We found that gulp-embed-json demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.