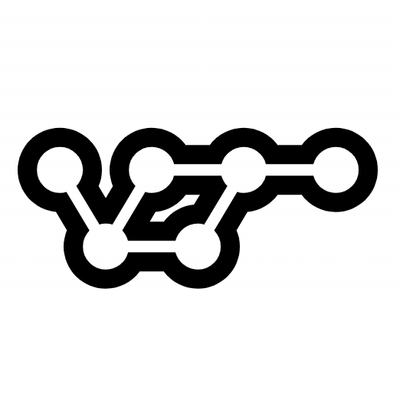
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
mirax-player
Advanced tools
Mirax Player is a simple video player for react web application.
To install the Mirax Player, you can use the following npm command:
npm install mirax-player
You can apply it in react app
In you React, app.js or main.js / jsx extension
add:
import 'mirax-player/mirax.css';
Then use the attach from Mirax Player:
import React, { useEffect, useState } from 'react';
import Mirax, { attach } from 'mirax-player';
const ExampleComponent = () => {
const [isPlaying, setIsPlaying] = useState(false);
useEffect(() => {
const videoElement = attach('.whatever');
// you can declare any variable here starts with . (point known as class selector)
// find className="whatever" src="clip.mp4"
const options = {
playPauseBtn: '.play-pause-btn',
volumeSlider: '.volume-slider',
progressBar: '.progress-bar',
currentTimeStamp: '.current-time',
durationTimeStamp: '.duration-time',
fullscreenBtn: '.fullscreen-btn',
};
const videoPlayer = new Mirax(videoElement, options);
// Listen for the "timeupdate" event to update time values
videoElement.addEventListener('timeupdate', () => {
videoPlayer.updateCurrentTimeAndDuration();
});
return () => {
// Clean up any resources if needed
};
}, []);
const togglePlayPause = () => {
setIsPlaying(prevIsPlaying => {
const video = attach('.whatever');
if (prevIsPlaying) {
video.pause();
} else {
video.play();
}
return !prevIsPlaying;
});
};
return (
<div className="video-player">
<video className="whatever" src="clip.mp4"></video>
<div className="controls">
<div className="volume-icon">
<div className="speaker"></div>
</div>
<button className="play-pause-btn" onClick={togglePlayPause}>
{isPlaying ? 'Pause' : 'Play'}
</button>
<input
type="range"
className="volume-slider"
min="0"
max="1"
step="0.01"
defaultValue="1"
/>
<progress className="progress-bar" min="0" max="100" value="0"></progress>
<div className="current-time"></div>
<div className="duration-time"></div>
<button className="fullscreen-btn">Fullscreen</button>
</div>
</div>
);
};
export default ExampleComponent;
MIT
npm install mirax-player
FAQs
A free video player compatible with React, Vue, Angular, and Svelte.
The npm package mirax-player receives a total of 0 weekly downloads. As such, mirax-player popularity was classified as not popular.
We found that mirax-player demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.