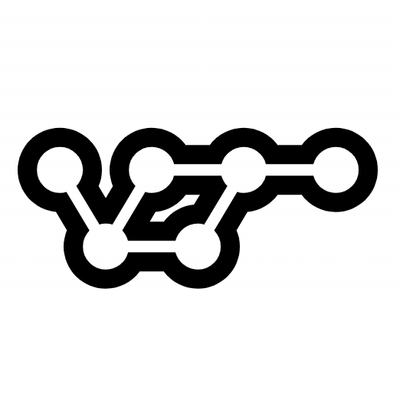
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
mirax-player
Advanced tools
Mirax Player is a video player has compatibility of typescript and javascript for React, Vue, Angular and Svelte.
Mirax Player is a video player has compatibility of typescript and javascript for React, Vue, Angular and Svelte. You can customize the theme color of the video player. Robust and easy to implement with readability syntax and light-weight.
To install the Mirax Player, you can use the following npm command:
npm install mirax-player
Keyboard keys / buttons | Functions | Description | Supported Browsers |
---|---|---|---|
space bar | Play & Pause | The video will play or pause | All browsers |
click ▶ | Play & Pause | The video will play or pause | All browsers |
alt+p or cmd+p | PiP | Picture in Picture screen | !firefox auto pip icon |
click Γ | PiP | Picture in Picture screen | All browsers |
double click the video | Fullscreen | It will set as fullscreen mode | All browsers |
click ❐ | Fullscreen | It will set as fullscreen mode | All browsers |
swipe for volume | Volume | To adjust the volume level | All browsers |
swipe for time frame | Progress bar | To adjust video frame timestamp | All browsers |
In your component
Then use it from Mirax Player:
//both react,vue, angular and svelte importing syntax
import { miraxplayer } from 'mirax-player';
// for react (video file stored: public/clip.mp4)
const video = useRef(null);
//
// for vue (video file stored: public/clip.mp4)
const video = ref(null);
//
// for angular (video file stored: src/assets/clip.mp4)
@ViewChild('video', { static: true })
//
// for svelte (video file stored: public/clip.mp4)
let video;
//
import React, { useEffect, useRef } from 'react';
import { miraxplayer } from 'mirax-player';
const ExampleComponent = () => {
const video = useRef(null);
useEffect(() => {
if (video.current) {
miraxplayer(video.current);
}
}, []);
return (
<div className='whatever'>
<video ref={video} className="mirax-player" src="clip.mp4"></video>
</div>
);
};
export default ExampleComponent;
import React, { useEffect, useRef } from 'react';
import { miraxplayer } from 'mirax-player';
const ExampleComponent: React.FC = () => {
const video = useRef<HTMLVideoElement>(null);
useEffect(() => {
if (video.current) {
miraxplayer(video.current);
}
}, []);
return (
<div className='whatever'>
<video ref={video} className="mirax-player" src="clip.mp4"></video>
</div>
);
};
export default ExampleComponent;
<template>
<div class="whatever">
<video ref="video" class="mirax-player" src="clip.mp4"></video>
</div>
</template>
<script>
import { ref, onMounted } from 'vue';
import { miraxplayer } from 'mirax-player';
export default {
name: 'ExampleComponent',
setup() {
const video = ref(null);
onMounted(() => {
if (video.value) {
miraxplayer(video.value);
}
});
return {
video
};
}
};
</script>
<template>
<div class="whatever">
<video ref="video" class="mirax-player" src="clip.mp4"></video>
</div>
</template>
<script lang="ts">
import { ref, onMounted } from 'vue';
import { miraxplayer } from 'mirax-player';
export default {
name: 'ExampleComponent',
setup() {
const video = ref<HTMLVideoElement | null>(null);
onMounted(() => {
if (video.value) {
miraxplayer(video.value);
}
});
return {
video
};
}
};
</script>
In your Angular component
import { Component, ElementRef, OnInit, ViewChild } from '@angular/core';
import { miraxplayer } from 'mirax-player';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent implements OnInit {
@ViewChild('video', { static: true }) video!: ElementRef<HTMLVideoElement>;
ngOnInit(): void {
this.initializeMirax();
}
initializeMirax() {
if (this.video.nativeElement) {
new miraxplayer(this.video.nativeElement).init();
}
}
}
<div>
<div class="whatever">
<video #video class="mirax-player" src="assets/clip.mp4"></video>
</div>
</div>
In your Svelte component
<script>
import { onMount } from 'svelte';
import { miraxplayer } from 'mirax-player';
let video;
onMount(() => {
if (video) {
miraxplayer(video);
}
});
</script>
<div class='whatever'>
<video bind:this={video} class="mirax-player" src="clip.mp4">
<track kind="captions" src="" label="English" default>
</video>
</div>
<script lang="ts">
import { onMount } from 'svelte';
import { miraxplayer } from 'mirax-player';
let video: HTMLVideoElement;
onMount(() => {
if (video) {
miraxplayer(video);
}
});
</script>
<div class='whatever'>
<video bind:this={video} class="mirax-player" src="clip.mp4">
<track kind="captions" src="" label="English" default>
</video>
</div>
// in React
<div className='whatever'>
<video ref={video} className="mirax-player" src="clip.mp4"></video>
</div>
// in Vue
<div class="whatever">
<video ref="video" class="mirax-player" src="clip.mp4"></video>
</div>
// in Angular
<div class="whatever">
<video #video class="mirax-player" src="assets/clip.mp4"></video>
</div>
// in Svelte
<div class='whatever'>
<video bind:this={video} class="mirax-player" src="clip.mp4">
<track kind="captions" src="" label="English" default>
</video>
</div>
.whatever {
margin: 0 auto;
position: relative;
width: 100%;
text-align: left;
}
.mirax-theme {
float: left!important;
background-color: rgba(36, 22, 223, 0.5)!important;
}
progress::-webkit-progress-value {
background-color: rgb(65, 7, 224, 0.9)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgb(65, 7, 224, 0.9)!important;
}
progress::-ms-fill {
background-color: rgb(65, 7, 224, 0.9)!important;
}
.whatever {
margin: 0 auto;
position: relative;
width: 100%;
text-align: center;
}
.mirax-theme {
margin: 0 auto!important;
background-color: rgba(36, 22, 223, 0.5)!important;
}
progress::-webkit-progress-value {
background-color: rgb(65, 7, 224, 0.9)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgb(65, 7, 224, 0.9)!important;
}
progress::-ms-fill {
background-color: rgb(65, 7, 224, 0.9)!important;
}
.whatever {
margin: 0 auto;
position: relative;
width: 100%;
text-align: right;
}
.mirax-theme {
float: right!important;
background-color: rgba(36, 22, 223, 0.5)!important;
}
progress::-webkit-progress-value {
background-color: rgb(65, 7, 224, 0.9)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgb(65, 7, 224, 0.9)!important;
}
progress::-ms-fill {
background-color: rgb(65, 7, 224, 0.9)!important;
}
.whatever {
margin: 0 auto;
position: relative;
width: 100%;
}
progress::-webkit-progress-value {
//change color here
}
progress[value]::-moz-progress-bar {
//change color here
}
progress::-ms-fill {
//change color here
}
-note always put !important at the end of statement.
.mirax-theme {
background-color: rgba(4, 88, 25, 0.2)!important;
}
progress::-webkit-progress-value {
background-color: rgb(252, 227, 7)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgb(252, 227, 7)!important;
}
progress::-ms-fill {
background-color: rgb(252, 227, 7)!important;
}
Transparency color mode:
Solid color mode:
.mirax-theme {
background: none !important;
}
Sample themes:
.mirax-theme {
background-color: purple!important;
}
progress::-webkit-progress-value {
background-color: lime!important;
}
progress[value]::-moz-progress-bar {
background-color: lime!important;
}
progress::-ms-fill {
background-color: lime!important;
}
.mirax-theme {
background-color: rgba(250, 149, 35, 0.9)!important;
}
progress::-webkit-progress-value {
background-color: rgb(17, 117, 59)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgb(17, 117, 59)!important;
}
progress::-ms-fill {
background-color: rgb(17, 117, 59)!important;
}
.mirax-theme {
background: none !important;
}
progress::-webkit-progress-value {
background-color: rgba(253, 75, 90, 0.897)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgba(253, 75, 90, 0.897)!important;
}
progress::-ms-fill {
background-color: rgba(253, 75, 90, 0.897)!important;
}
.mirax-theme {
background: none !important;
}
progress::-webkit-progress-value {
background-color: rgba(250, 234, 5, 0.7)!important;
}
progress[value]::-moz-progress-bar {
background-color: rgba(250, 234, 5, 0.7)!important;
}
progress::-ms-fill {
background-color: rgba(250, 234, 5, 0.7)!important;
}
MIT
Demjhon Silver
FAQs
A free video player compatible with React, Vue, Angular, and Svelte.
The npm package mirax-player receives a total of 0 weekly downloads. As such, mirax-player popularity was classified as not popular.
We found that mirax-player demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.