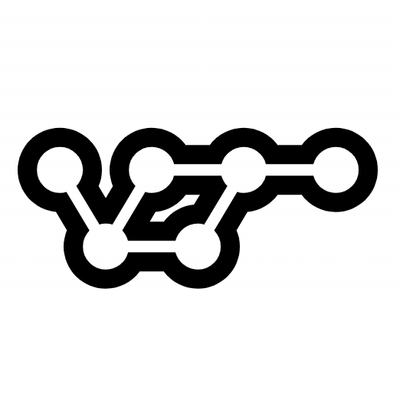
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Netmorphic is a library for testing networked applications, such as REST clients over HTTP, and database clients over TCP.
The easiest way to get started is to use the netmorphic template application.
git clone https://github.com/ql-io/netmorphic.git
cd netmorphic
make clean install
npm install netmorphic
var netmorphic = require('netmorphic')
Configure your proxy with a json file. See the examples below.
RESTful routing is made available through the use of the Router module.
{
"/path/to/endpoint":{ // this is static url for the endpoint
"host":"endpoint.host.example.com", // the host of the endpoint server
"port":80, // the port of the endpoint
"type":"slow", // the handler type to use. See section on handlers below
"method":"get", // request method
"latency": 100 // latency parameter to use with "slow" handler type
},
"/product/{id}":{ // this is a dynamic url. The value of id will be found at req.params.id
"host":"endpoint.host.example.com",
"port":80,
"type":"flakey", // another type of handler
"method":"get",
"hi" : 2000,
"lo" : 500,
},
"addresses" : [] // leave this empty if you don't need to bother with multi-tenancy
}
TCP configuration is similar to the above, with two major exceptions. The first is that multi-tenancy is not currently supported, so there is no 'global' key at the top level. The second is that urls are replaced with the the port number that the proxy server will listen on.
{
"10001": { // the port the proxy will listen on
"host" : "127.0.0.1", // and proxy incoming streams to this host
"port" : 3124, // and this port
"type" : "normal" // using this TCP handler
},
"10002": { // use this port for a slow proxy
"host" : "127.0.0.1",
"port" : 3124,
"type" : "slow",
"latency" : 5000
},
"10003": { // use this port for a drop proxy
"host" : "127.0.0.1",
"port" : 3124,
"type" : "drop",
"lo" : 1000,
"high" : 5000
},
"10004": { // use this port for a bumpy proxy
"host" : "127.0.0.1",
"port" : 3124,
"type" : "bumpy",
"lo" : 3500,
"high" : 7000
}
}
var netmorphic = require('netmorphic').http
, config = require('files/myconfig.json')
, USE_CLUSTER = false
, CUSTOM_HANDLERS = false;
var proxy = netmorphic(CONFIG, CUSTOM_HANDLERS, USE_CLUSTER);
proxy.server.listen(8000)
var netmorphic = require('netmorphic').http
, monitor = require('netmorphic').monitor
, Cluster = requir('cluster2')
, config = require('files/myconfig.json')
, USE_CLUSTER = true
, CUSTOM_HANDLERS = false;
var proxy = netmorphic(CONFIG, CUSTOM_HANDLERS, USE_CLUSTER);
var cluster = new Cluster({
port: 8000,
monitor: monitor()
})
cluster.listen(function(cb){
cb(proxy.server)
})
var TCProxy = require('netmorphic').tcp
, config = require('files/TCP.config.json')
, CUSTOM_HANDLERS = false
, USE_CLUSTER = false;
// returns an array of servers
var servers = TCProxy(config, CUSTOM_HANDLERS, USE_CLUSTER)
//iterate over the TCP servers and start each one
servers.forEach(function(server){
server.app.listen(server.port)
})
var TCProxy = require('netmorphic').tcp
, monitor = require('netmorphic').monitor
, config = require('files/TCP.config.json')
, Cluster = require('cluster2')
, CUSTOM_HANDLERS = false
, USE_CLUSTER = true;
var servers = TCProxy(config, CUSTOM_HANDLERS, USE_CLUSTER)
var cluster = new Cluster({
monitor: monitor()
})
cluster.listen(function(cb){
cb(servers)
})
Handlers are are the "morph" in netmorphic. They act upon your requests and streams. Parameters for your handlers are set in the config files. Netmorphic ships with a few handlers out of the box, namely:
Additionally, custom handlers can be written to do anything. Pass an object of handler functions to the netmorphic constructor, like so:
var TCProxy = require('netmorphic').tcp
, config = require('files/TCP.config.json')
, CUSTOM_HANDLERS = require('files/my.custom.handlers.js')
, USE_CLUSTER = true;
var servers = TCProxy(config, CUSTOM_HANDLERS, USE_CLUSTER)
a custom HTTP handler file would look like this:
// hint: it's just a function that handles the request and response streams...
module.exports['just proxy'] = function(req, res){
var config = req.serConfig; // the service config for this particular client
var proxy = req.proxy; // a proxy to use, if you need a proxy
proxy.proxyRequest(req, res, {
host:config.host,
port:config.port
});
}
For TCP, it looks like this:
var ps = require('pause-stream'); // a stream that pauses
module.exports['vanilla tcp proxy'] = function(socket, service){
// socket is the client stream
// service is a TCP socket to use to proxy to the endpoint
var buffer = ps(); // create a pause stream
socket.pipe(buffer.pause()); // pipe the request to the buffer and pause it
service.connect(socket._CONFIG.port, socket._CONFIG.host, function(){
buffer.pipe(service); // pipe the buffered request to the endpoint
buffer.resume() // resume the buffer stream
});
service.pipe(socket); // pipe the endpoint connection back to the client connection
}
FAQs
ERROR: No README.md file found!
The npm package netmorphic receives a total of 1 weekly downloads. As such, netmorphic popularity was classified as not popular.
We found that netmorphic demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.