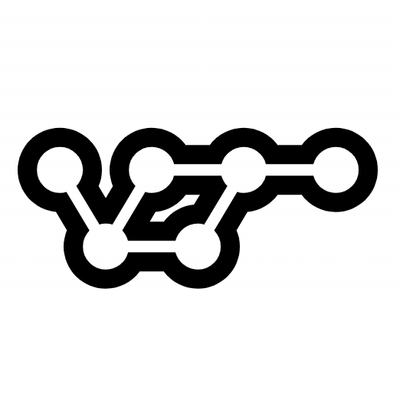
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ng-teximate
Advanced tools
A simple module for CSS3 text animations | live demo
Install it with npm
npm install ng-teximate --save
If you are using SystemJS, you should also adjust your configuration to point to the UMD bundle.
In your systemjs config file, map
needs to tell the System loader where to look for ng-teximate
:
map: {
'ng-teximate': 'node_modules/ng-teximate/bundles/ng-teximate.umd.js',
}
Here is a working plunker
Import TeximateModule in your root module
import {TeximateModule} from "ng-teximate";
@NgModule({
imports: [
TeximateModule
]
})
Teximate uses animate.css to animate words/letters.
install it npm install animate.css --save
and in your global style import it
/* You can add global styles to this file, and also import other style files */
@import '~animate.css';
or import it using the CDN
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/animate.css/3.5.2/animate.min.css" />
<teximate #teximate [text]="text" [type]="type" [effect]="options"></teximate>
export class SomeComponent {
text = 'It’s kind of fun to do the impossible. 👾';
options: TeximateOptions = {
animation: { name: 'zoomInLeft', duration: 1000 },
word: { type: TeximateOrder.SHUFFLE, delay: 100 },
letter: { type: TeximateOrder.SHUFFLE, delay: 50 }
};
type = 'letter';
// Create another effect without
@ViewChild(TeximateComponent) teximate: TeximateComponent;
ngOnInit(){
const diffOptions: TeximateOptions = {
animation: { name: 'bounce', duration: 1000 },
word: { type: TeximateOrder.SEQUENCE, delay: 100 },
letter: { type: TeximateOrder.SEQUENCE, delay: 50 }
};
setTimeout(()=>{
this.teximate.runEffect(diffOptions, 'letter');
}, 2500);
}
}
Most often you won't need to use ViewChild
and call runEffect
because you can run the effect automatically by changing inputs.
You can also access any line, word or letter by by its class, for example you can apply the following css rules in the global style.css
.letter{
text-shadow: 1px 1px 1px rgba(#000, .5);
}
.word1{
background-color: red;
}
.letter2{
color: blue;
}
NOTE that this won't effect if you add the from your component style unless you activate ViewEncapsulation.None
on it.
What else? Support this module by giving it a star, this will help it to get updates and fixes more frequently
If you identify any errors in this module, or have an idea for an improvement, please open an issue. I am excited to see what the community thinks of this project, and I would love your input!
FAQs
A simple module for CSS3 text animations.
The npm package ng-teximate receives a total of 3 weekly downloads. As such, ng-teximate popularity was classified as not popular.
We found that ng-teximate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.