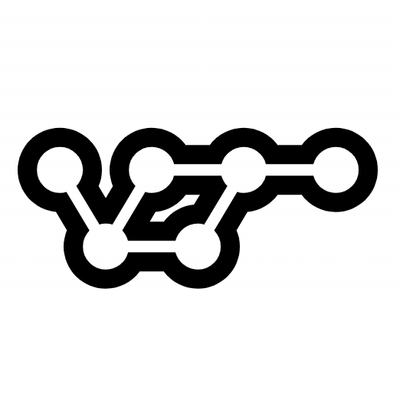
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ng2-inline-svg
Advanced tools
Angular 2 directive for inserting an SVG inline within an element, allowing for easily styling
with CSS like fill: currentColor;
.
This is based on md-icon, except this is meant purely for inserting SVG files within an element, without the extra things like font icons.
npm install --save ng2-inline-svg
Make sure to add HTTP_PROVIDERS
to your list of bootstrap providers:
import { bootstrap } from '@angular/platform-browser-dynamic';
import { HTTP_PROVIDERS } from '@angular/http';
import { App } from './app/app.component';
bootstrap(App, [HTTP_PROVIDERS]);
Import the component and add it to the list of directives on your component:
import { Component } from '@angular/core';
import InlineSVG from 'ng2-inline-svg';
@Component({
selector: 'app',
directives: [InlineSVG],
template: `
<div class="my-icon" aria-label="My icon" [inlineSVG]="'/img/image.svg'"></div>
`
})
export class App {
}
The SVG file (if found) will be inserted inside the element with the [inlineSVG]
directive.
[cacheSVG]
Caches the SVG based on the absolute URL. Cache only persists for the (sessional) lifetime of the page.
Default: true
[replaceContents]
Replaces the contents of the element with the SVG instead of just appending it to its children.
Default: true
(onSVGInserted)
Emits the SVGElement
that was inserted.
FAQs
[DEPRECATED] Renamed to ng-inline-svg.
The npm package ng2-inline-svg receives a total of 7 weekly downloads. As such, ng2-inline-svg popularity was classified as not popular.
We found that ng2-inline-svg demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.