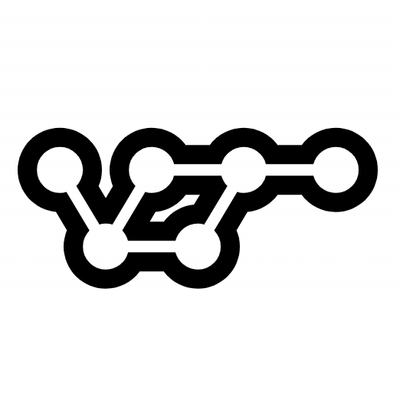
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ng2-pdf-viewer
Advanced tools
ng2-pdf-viewer is an Angular component for viewing PDF files. It provides a simple way to display PDF documents in your Angular applications with various customization options.
Basic PDF Viewing
This feature allows you to display a PDF file in your Angular application. The 'src' attribute specifies the path to the PDF file, and 'render-text' enables text rendering.
<pdf-viewer [src]="'path/to/pdf-file.pdf'" [render-text]="true"></pdf-viewer>
Pagination
This feature allows you to navigate through the pages of a PDF document. The 'page' attribute specifies the current page, and you can use buttons to change the page.
<pdf-viewer [src]="'path/to/pdf-file.pdf'" [page]="page"></pdf-viewer>
<button (click)="page = page - 1">Previous</button>
<button (click)="page = page + 1">Next</button>
Zoom
This feature allows you to zoom in and out of the PDF document. The 'zoom' attribute specifies the zoom level, and you can use buttons to adjust the zoom level.
<pdf-viewer [src]="'path/to/pdf-file.pdf'" [zoom]="zoom"></pdf-viewer>
<button (click)="zoom = zoom + 0.1">Zoom In</button>
<button (click)="zoom = zoom - 0.1">Zoom Out</button>
Rotation
This feature allows you to rotate the PDF document. The 'rotation' attribute specifies the rotation angle, and you can use a button to rotate the document by 90 degrees.
<pdf-viewer [src]="'path/to/pdf-file.pdf'" [rotation]="rotation"></pdf-viewer>
<button (click)="rotation = rotation + 90">Rotate</button>
Text Layer
This feature enables the text layer, which makes the text in the PDF selectable and searchable. The 'render-text' attribute is set to true to enable this feature.
<pdf-viewer [src]="'path/to/pdf-file.pdf'" [render-text]="true"></pdf-viewer>
pdfjs-dist is a library that provides a low-level API to render PDF documents using JavaScript. It is more flexible and powerful but requires more effort to integrate into an Angular application compared to ng2-pdf-viewer.
ngx-extended-pdf-viewer is another Angular component for viewing PDF files. It offers more features and customization options than ng2-pdf-viewer, such as support for forms, annotations, and a built-in toolbar.
react-pdf is a React component for viewing PDF files. While it is not for Angular, it offers similar functionalities to ng2-pdf-viewer for React applications, including basic viewing, pagination, and zooming.
PDF Viewer Component for Angular 2
npm install ng2-pdf-viewer --save
In your system.config.js
Append to map
var map = {
...
'ng2-pdf-viewer': 'node_modules/ng2-pdf-viewer'
}
and then add to packages
var packages = {
...
'ng2-pdf-viewer': { main: 'dist/pdf-viewer.component.min.js' }
}
Import component to your component
import { Component } from '@angular/core';
import { PdfViewerComponent } from 'ng2-pdf-viewer';
@Component({
selector: 'example-app',
template: `
<div>
<label>PDF src</label>
<input type="text" placeholder="PDF src" [(ngModel)]="pdfSrc">
</div>
<div>
<label>Page:</label>
<input type="number" placeholder="Page" [(ngModel)]="page">
</div>
<pdf-viewer [src]="pdfSrc" [initialPage]="page" [original-size]="true" style="display: block;"></pdf-viewer>
`,
directives: [PdfViewerComponent]
})
export class AppComponent {
pdfSrc: string = '/pdf-test.pdf';
page: number = 1;
}
Pass pdf location
[src]="https://vadimdez.github.io/ng2-pdf-viewer/pdf-test.pdf"
Page number
[page]="1"
if set to true - size will be as same as original document
if set to false - size will be as same as container block
[original-size]="true"
Show single or all pages altogether
[show-all]="true"
0.0.6
FAQs
Angular 5+ component for rendering PDF
The npm package ng2-pdf-viewer receives a total of 131,213 weekly downloads. As such, ng2-pdf-viewer popularity was classified as popular.
We found that ng2-pdf-viewer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.