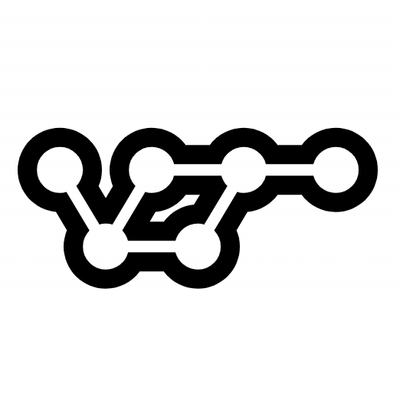
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ng6-popup-boxes
Advanced tools
NOTE : Tested with Angular v6.0.0 and Angular-Cli v6.0.0.
Install ng6-popup-boxes using npm:
$ npm install ng6-popup-boxes --save
Add PopupBoxesModule into your AppModule class. app.module.ts would look like this: Take Note : For Toastr Animations working perfectly please import BrowserAnimationsModule into app.module.ts file.
import { BrowserModule } from '@angular/platform-browser';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { PopupBoxesModule } from 'ng6-popup-boxes';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, BrowserAnimationsModule, PopupBoxesModule.forRoot()],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
Inject 'PopupManager' class in your component class.
import { Component } from '@angular/core';
import { PopupManager } from './ng6-popup-boxes';
import { LoadingComponent } from './loading/loading.component';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
constructor(public popupManager: PopupManager) {}
showAlertBox() {
this.popupManager.open(
'Alert Box',
"Let's go up in here, and start having some fun The very fact that you're aware of suffering is enough reason to be overjoyed that you're alive and can experience it. It's a super day, so why not make a beautiful sky? ",
{
width: '300px',
popupClass: 'my-popup-box',
animate: 'slide-from-top',
showOverlay: true,
position: 'top',
callback: (result: any) => {
if (result) {
this.customDialog('You clicked Ok');
} else {
this.customDialog('You clicked Cancel');
}
}
}
);
}
customDialog(message: any) {
this.popupManager.open('Custom Dialog', message, {
width: '300px',
position: 'bottom',
animate: 'scale',
actionButtons: [
{
text: 'Done',
buttonClasses: 'btn-ok'
}
]
});
}
showConfirmBox() {
this.popupManager.open('Delete Confirmation', 'Do you really want to this item?', {
width: '300px',
closeOnOverlay: false,
animate: 'scale',
actionButtons: [
{
text: 'Yes',
buttonClasses: 'btn-ok',
onAction: () => {
return true;
}
},
{
text: 'No',
buttonClasses: 'btn-cancel',
onAction: () => {
return false;
}
}
],
callback: (result: any) => {
if (result) {
this.showLoadingBox();
}
}
});
}
showLoadingBox() {
let popup = this.popupManager.open('', '', {
width: '250px',
injectComponent: LoadingComponent,
showTitle: false,
showMessage: false,
showCloseBtn: false,
closeOnOverlay: false,
animate: 'slide-from-bottom',
actionButtons: [],
callback: (result: any) => {
if (result) {
this.deleteSuccessBox();
}
}
});
// some async call & then close
setTimeout(() => {
popup.close(true);
}, 2000);
}
deleteSuccessBox() {
this.popupManager.open('Success', 'Record has been deleted successfully !', {
width: '300px',
animate: 'slide-from-top',
position: 'top',
actionButtons: [
{
text: 'Done',
buttonClasses: 'btn-ok'
}
]
});
}
}
actionButtons: [
{
text: 'Cancel',
buttonClasses: 'btn-cancel',
onAction: () => {
return false;
}
},
{
text: 'Ok',
buttonClasses: 'btn-ok',
onAction: () => {
return true;
}
}
]
callback: (result: any) => {
if (result) {
alert('You clicked Ok');
} else {
alert('You clicked Cancel');
}
}
[Ng6 Popup Demo Link] (http://technoidlab.com/demos/ng6-popup-boxes)
$ cd demo/ngcli && npm install
$ ng serve
Then navigate your browser to http://localhost:4200
$ cd demo/webpack && npm run install
$ npm run build
$ npm start
FAQs
NOTE : Tested with Angular v6.0.0 and Angular-Cli v6.0.0.
The npm package ng6-popup-boxes receives a total of 2 weekly downloads. As such, ng6-popup-boxes popularity was classified as not popular.
We found that ng6-popup-boxes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.