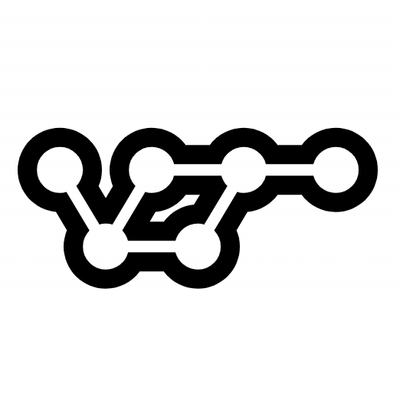
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
ngx-avatar
Advanced tools
[](https://travis-ci.org/HaithemMosbahi/ngx-avatar)
A universal avatar component for Angular 2+ applications that fetch / generates avatar based on the informations you have about the user. The component has a fallback system that if for example an invalid Facebook ID is used it will try google ID and so on.
You can use this component whether you have a single source or a multiple avatar sources. In this case the fallback system will fetch the first valid avatar.
Moreover, the component can shows name initials or simple value as avatar.
Supported avatar sources:
The fallback system uses the same order as the above source list, Facebook has the highest priority, if it fails, google source will be used, and so on.
Install avatar component using NPM::
$ npm install ngx-avatar --save
Or download as ZIP.
Once you have installed ngx-avatar, you can import it in your AppModule
:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
// Import your AvatarModule
import { AvatarModule } from 'ngx-avatar';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
// Specify AvatarModule as an import
AvatarModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Once the AvatarModule is imported, you can start using the component in your Angular application:
<ngx-avatar></ngx-avatar>
<ngx-avatar facebookId="1508319875"></ngx-avatar>
<ngx-avatar googleId="1508319875"></ngx-avatar>
<ngx-avatar twitterId="1508319875"></ngx-avatar>
<ngx-avatar name="Jhon Doe"></ngx-avatar>
<ngx-avatar value="75%"></ngx-avatar>
<ngx-avatar source="facebook" skypeId="skype" googleId="google" name="Haithem Mosbahi" custom="assets/custom.jpg" value="28%" initials facebookId="100008343750912" twitterId="twitter" gravatarId="gravatar" size="100" [round]="true"></ngx-avatar>
Attribute | Type | Default | Description |
---|---|---|---|
facebook-id | string | ||
twitter-id | string | ||
google-id | string | ||
skype-id | string | ||
name | string | Will be used to generate avatar based on the initials of the person | |
value | string | Show a value as avatar | |
color | string | random | Give the background a fixed color with a hex like for example #FF0000 |
fgColor | string | #FFF | Used in combination with name and value . Give the text a fixed color with a hex like for example #FF0000 |
size | number | 50 | Size of the avatar |
textSizeRatio | number | 3 | For text based avatars the size of the text as a fragment of size (size / textSizeRatio) |
round | bool | true | Round the avatar corners |
src | string | Fallback image to use | |
style | object | Style that will be applied on the root element |
To generate all *.js
, *.d.ts
and *.metadata.json
files:
$ npm run build
To lint all *.ts
files:
$ npm run lint
MIT © Haithem Mosbahi
FAQs
A universal avatar component for Angular applications that fetches / generates avatar based on the information you have about the user.
The npm package ngx-avatar receives a total of 4,482 weekly downloads. As such, ngx-avatar popularity was classified as popular.
We found that ngx-avatar demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.