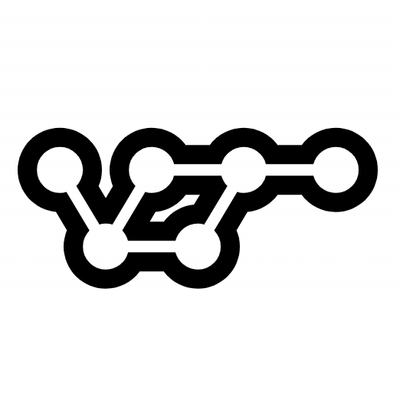
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
node-cipher
Advanced tools
Securely encrypt sensitive files for use in public source control. Find on NPM.
Why would I want to encrypt my files?
Let's say you have a file in your project name config.json
which contains sensitive information like private keys and database passwords.
What happens if you want to publicly host a repository containing this file? Certainly you wouldn't want to make the contents of config.json
visible to the outside world, so instead you can use node-cipher to encrypt the file and add its encrypted counterpart to source control, which can later be decrypted using the encryption key when the repository is cloned.
Just don't forget to add the original config.json
file to .gitignore
!
$ npm install -g node-cipher
Looking for the Node JS API? Click here.
$ nodecipher [--list] <command> -i input -o output [-p password] [-a algorithm]
When in doubt, $ nodecipher --help
Command | Description |
---|---|
encrypt | Encrypts a file using the arguments provided. |
decrypt | Decrypts a file using the arguments provided. |
Flag | Alias | Description | Required | Default |
---|---|---|---|---|
input | i | The input filename relative to the current working directory. | ✓ | |
output | p | The output filename relative to the current working directory. | ✓ | |
password | p | The key that you will use to encrypt or decrypt your file. If this is not supplied directly, you will instead be prompted within your command line. If you are decrypting a file, the password must be the same as the one specified during encryption, or else the decryption will fail. | ||
algorithm | a | The cipher algorithm that you will use to encrypt or decrypt your file. If you are decrypting a file, the chosen algorithm must be the same as the one specified during encryption, or else the decryption will fail. | cast5-cbc | |
list | l | Lists all available cipher algorithms. | ||
version | v | Show the node-cipher version. | ||
help | h | Show the help menu. |
Encrypts config.json
into config.encrypted.json
using the aes-128-cbc
cipher algorithm.
$ nodecipher encrypt -i "config.json" -o "config.encrypted.json" -a aes-128-cbc
Looking for the CLI? Click here.
Name | Type | Description | Required | Default |
---|---|---|---|---|
input | string | The input filename relative to the current working directory. | ✓ | |
output | string | The output filename relative to the current working directory. | ✓ | |
password | string | The encryption password. Unlike the command line interface, this MUST be specified. | ✓ | |
algorithm | string | The algorithm to use. Use nodecipher -l to see a list of available cipher algorithms. | "cast5-cbc" |
encrypt(options[, callback[, scope]])
Encrypt a file using the options provided.
Parameter | Type | Description | Required |
---|---|---|---|
options | Object | The NodeCipher options Object. | ✓ |
callback | Function | The function to call when the encryption has completed. | |
scope | Object | The Function scope for the callback parameter, if provided. |
Encrypts config.json
into config.encrypted.json
using the password "b0sco"
.
let nodecipher = require('node-cipher');
nodecipher.encrypt({
input: 'config.json',
output: 'config.encrypted.json',
password: 'b0sco'
}, function (err) {
if (err) throw err;
console.log('config.json encrypted.');
});
decrypt(options[, callback[, scope]])
Decrypts a file using the options provided.
Parameter | Type | Description | Required |
---|---|---|---|
options | Object | The NodeCipher options Object. | ✓ |
callback | Function | The function to call when the decryption has completed. | |
scope | Object | The Function scope for the callback parameter, if provided. |
Decrypts config.encrypted.json
back into config.json
using the password "b0sco"
.
let nodecipher = require('node-cipher');
nodecipher.decrypt({
input: 'config.encrypted.json',
output: 'config.json',
password: 'b0sco'
}, function (err) {
if (err) throw err;
console.log('config.encrypted.json decrypted.');
});
list():Array
Lists all available cipher algorithms as an Array.
let nodecipher = require('node-cipher');
console.log(nodecipher.list());
// => ['CAST-cbc', 'aes-128-cbc', ..., 'seed-ofb']
Using NPM, you can create custom scripts in our package.json
file to automate much of the encryption/decryption process.
{
// ...
"scripts": {
"encrypt": "nodecipher encrypt -i config.json -o config.encrypted.json",
"decrypt": "nodecipher decrypt -i config.encrypted.json -o config.json"
}
}
Simply run npm run encrypt
or npm run decrypt
to execute these commands.
MIT
FAQs
Securely encrypt sensitive files for use in public source control.
The npm package node-cipher receives a total of 307 weekly downloads. As such, node-cipher popularity was classified as not popular.
We found that node-cipher demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.