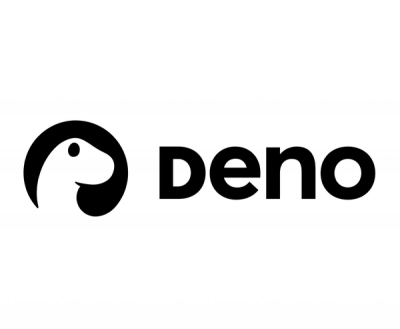
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
SDK for pay.nl allowing you to manage your pay.nl transactions in nodeJS
npm install paynl-sdk --save
var Paynl = require('paynl-sdk');
Paynl.Config.setApiToken('Your-api-token');
Paynl.Config.setServiceId('SL-0123-4567');
Some of the basic examples are listed here, for the full list of examples, please take a look at the samples directory here
All examples start with requiring paynl-sdk and setting the apitoken and serviceId.
var Paynl = require('paynl-sdk');
Paynl.Config.setApiToken('Your-api-token');
Paynl.Config.setServiceId('SL-0123-4567');
This example shows how to fetch a list of the available payment methods.
The method Paynl.Paymentmethods.getList()
returns an observable array.
For more information about observables see reactivex.io
Paynl.Paymentmethods.getList().forEach(
function(paymentmethod) {
console.log(paymentmethod.id + ' ' + paymentmethod.visibleName);
}
)
.catch(error => {
console.error(error)
});
This example shows the minimum required attributes to start a transaction. The full version with all supported options is located here
Paynl.Transaction.start({
//the amount in euro
amount: 19.95,
//we redirect the user back to this url after the payment
returnUrl: "https://my-return-url.com/return",
//the ip address of the user
ipAddress: '10.20.30.40'
})
.subscribe(
function (result) {
//redirect the user to this url to complete the payment
console.log(result.paymentURL);
// the transactionId, use this to fetch the transaction later
console.log(result.transactionId);
},
function (error) {
console.error(error);
}
);
The following example shows how to fetch a transaction.
Paynl.Transaction.get('715844054X85729e').subscribe(
function(result){
// some examples of what you can do with the result
if (result.isPaid()) {
console.log('The transaction is paid');
// refund a part of the transaction
result.refund({
amount: 0.5,
description: '50 cents refund'
});
}
if (result.isCanceled()) {
console.log('Tranasaction is canceled, restock the items');
}
if (result.isBeingVerified()) {
console.log('Transaction needs to be verified first, possible fraud');
result.decline(); //decline the transaction
result.approve(); //approve the transaction
}
},
function(error){
console.error(error);
}
);
Before you can send a transaction, you need to know which terminal to send the transaction to.
Paynl.Instore.getTerminals()
.forEach(function (terminal) {
console.log(terminal.id + ' ' + terminal.name);
})
.catch(function (error) { return console.error(error); });
First you need to start a transaction. After the transaction is started and you have a transactionId, you can send the transaction to the terminal. You can get the terminalId from the example above.
var lastStatus = null;
Paynl.Instore.payment(transactionId, terminalId).subscribe(
//this gets called every 3 seconds
status => {
//save the last status so we can access it in the complete function
lastStatus = status;
console.log(status.state + ' Percentage: ' + status.percentage);
},
error => console.trace(error),
() => {
// if the transaction has reached a final state this function gets called
if (lastStatus.state == 'approved') {
// fetch the receipt of the transaction
Paynl.Instore.getReceipt(hash).subscribe(receipt => {
console.log(receipt.receipt);
}, error => {
console.trace(error);
});
} else {
console.log('Payment was not completed');
}
}
);
FAQs
Manage transactions in your PAY. account with nodejs
The npm package paynl-sdk receives a total of 655 weekly downloads. As such, paynl-sdk popularity was classified as not popular.
We found that paynl-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.