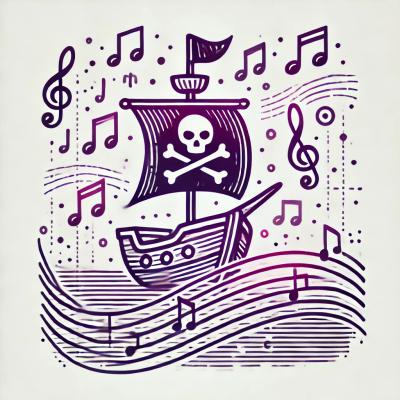
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
powerglove
Advanced tools
npm install powerglove
[(a -> *)] -> a -> Promise -> *
Passes a value through an array of functions sequentially; Returns value fulfilled from final function in the array.
Example:
import { pipe } from 'powerglove'
void async () => {
const weirdMath = pipe([
n => n / 1,
n => n + 4,
n => n * 7
])
await weirdMath(2)
// -> 42
}()
Params:
Type | Name | Description |
---|---|---|
Function[] | funcs | Array of functions |
* | x | Any value |
Return:
Type | Description |
---|---|
Promise | Fulfills with value returned by last index of func |
[(a -> *)] -> a -> Promise -> [*]
Executes an array of functions concurrently; returns an array of fulfilled values.
Example:
import { all } from 'powerglove'
void async () => {
const sayHi = all([
x => `Hello, ${x}!`,
x => `¡Hola, ${x}!`,
x => `Bonjour, ${x}!`
])
await sayHi('world')
// -> [ 'Hello, world!', '¡Hola, world!', 'Bonjour, world!' ]
}()
Params:
Type | Name | Description |
---|---|---|
Function[] | funcs | Array of functions |
* | x | Any value |
Return:
Type | Description |
---|---|
Promise | Fulfills with an array of values returned from each function in funcs |
[(a -> *)] -> a -> Promise -> *
Executes an array of functions concurrently; Returns value of first function to resolve.
Example:
import { race, delay } from 'powerglove'
void async () => {
const fast = delay(200)(x => `${x} Speed Racer`)
const faster = delay(100)(x => `${x} Racer X`)
const fastest = delay(0)(x => `${x} Chim Chim`)
const announceWinner = race([
fast,
faster,
fastest
])
await announceWinner('And the winner is...')
// -> `And the winner is... Chim Chim!`
}()
Params:
Type | Name | Description |
---|---|---|
Function[] | funcs | Array of functions |
* | x | Any value |
Return:
Type | Description |
---|---|
Promise | Fulfills with value of first function in funcs to resolve |
(a -> Bool) -> (a -> *) -> a -> Promise -> *
Executes function until done
returns true
. The return value of
the repeating function is passed into itself on each successive iteration.
Example:
import { until } from 'powerglove'
void async () => {
const minusminus = x => x - 1
const smallEnough = x => x <= 0
const subtractAll = until(smallEnough)(minusminus)
await subtractAll(100000)
// -> 0
const plusplus = x => x + 1
const largeEnough = x => x >= 100000
const addALot = until(largeEnough)(plusplus)
await addALot(0)
// -> 100000
}()
Params:
Type | Name | Description |
---|---|---|
Function | done | Accepts value returned by f . f is called repeatedly until this function returns true |
Function | f | Function to be called repeatedly. Passes its own return value into itself on each iteration |
* | x | Any value |
Return:
Type | Description |
---|---|
Promise | Fulfills result of f after n recursive calls |
(a -> Bool) -> (a -> *) -> (a -> *) -> a -> Promise -> *
Tests a value and passes value to either the pass
function or the fail
function
Example:
import { when } from 'powerglove'
void async () => {
const over9000 = lvl => lvl > 9000
const praise = lvl => `Holy crap! ${lvl}?! THAT'S OVER 9000!`
const insult = lvl => `Pffft. ${lvl}? Is that all you got???`
const analyzePowerLevel = when(over9000)(praise)(insult)
await analyzePowerLevel(9001)
// -> `Holy crap! 9001?! THAT'S OVER 9000!`
await analyzePowerLevel(1)
// -> `Pffft. 1? Is that all you got???`
}()
Params:
Type | Name | Default | Description |
---|---|---|---|
Function | test | Accepts x ; returns true or false | |
Function | pass | Called with x if test returns true | |
Function | fail | a => a | Called with x if test returns false |
* | x | Any value |
Return:
Type | Description |
---|---|
Promise | Fulfills with value returned by pass or fail |
Number -> (a -> *) -> a -> Promise -> *
Accepts ms
, number of milliseconds to wait, before executing function f
.
Example:
import { delay } from 'powerglove'
void async () => {
const timeSince = delay(300)(ms => Date.now() - ms)
await timeSince(Date.now())
// -> ~300ms
}()
Params:
Type | Name | Description |
---|---|---|
Number | funcs | Array of functions |
Function | f | Function to be called after timeout |
* | x | Any value |
Return:
Type | Description |
---|---|
Promise | Fulfills with value returned by f(x) |
FAQs
Async generator-based control flow
We found that powerglove demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.