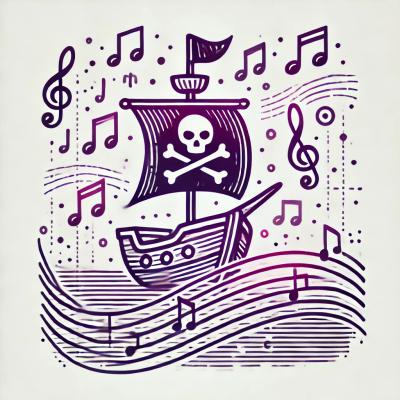
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
promise-thunk
Advanced tools
ES6 Promise + Thunk = PromiseThunk!
promise-thunk
is standard ES6 Promise implementation + thunk.
it supports browsers and node.js.
it throws unhandled rejection error.
for node.js:
$ npm install promise-thunk --save
or
for browsers:
https://lightspeedworks.github.io/promise-thunk/promise-thunk.js
<script src="https://lightspeedworks.github.io/promise-thunk/promise-thunk.js"></script>
you can use PromiseThunk.
in node.js
var PromiseThunk = require('promise-thunk');
in browsers
(function (PromiseThunk) {
'use strict';
// you can use PromiseThunk
})(this.PromiseThunk || require('promise-thunk'));
or
var PromiseThunk = this.PromiseThunk || require('promise-thunk');
var PromiseThunk = require('promise-thunk');
function sleepNodeStyle(msec, val, cb) {
console.log(val + ' timer start: ' + msec);
setTimeout(function () {
console.log(val + ' timer end : ' + msec);
cb(null, val);
}, msec);
}
var sleepPromiseThunk = PromiseThunk.wrap(sleepNodeStyle);
// promise type
sleepPromiseThunk(1000, 'a1')
.then(
function (val) {
console.log('a2 val: ' + val);
return sleepPromiseThunk(1000, 'a2'); },
function (err) { console.log('err:' + err); })
.catch(
function (err) { console.log('err:' + err); }
);
// thunk type
sleepPromiseThunk(1000, 'b1')
(function (err, val) {
console.log('b2 val: ' + val + (err ? ' err: ' + err : ''));
return sleepPromiseThunk(1000, 'b2'); })
(function (err, val) {
console.log('b3 val: ' + val + (err ? ' err: ' + err : '')); });
// mixed promise and thunk type
sleepPromiseThunk(1000, 'c1')
(function (err, val) {
console.log('c2 val: ' + val + (err ? ' err: ' + err : ''));
return sleepPromiseThunk(1000, 'c2'); })
.then(
function (val) {
console.log('c3 val: ' + val);
return sleepPromiseThunk(1000, 'c3'); },
function (err) { console.log('err:' + err); });
//output:
// a1 timer start: 1000
// b1 timer start: 1000
// c1 timer start: 1000
// a1 timer end : 1000
// a2 val: a1
// a2 timer start: 1000
// b1 timer end : 1000
// b2 val: b1
// b2 timer start: 1000
// c1 timer end : 1000
// c2 val: c1
// c2 timer start: 1000
// a2 timer end : 1000
// b2 timer end : 1000
// b3 val: b2
// c2 timer end : 1000
// c3 val: c2
// c3 timer start: 1000
// c3 timer end : 1000
how to make promise.
p = new PromiseThunk(
function setup(resolve, reject) {
// async process -> resolve(value) or reject(error)
try { resolve('value'); }
catch (error) { reject(error); }
}
);
// setup(
// function resolve(value) {},
// function reject(error) {})
example
var p = new PromiseThunk(
function setup(resolve, reject) {
setTimeout(function () {
if (Math.random() < 0.5) resolve('value');
else reject(new Error('error'));
}, 100);
}
);
// promise
p.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); });
// thunk
p(function (err, val) { console.info('val:', val, 'err:', err); });
// mixed chainable
p(function (err, val) { console.info('val:', val, 'err:', err); })
(function (err, val) { console.info('val:', val, 'err:', err); })
(function (err, val) { console.info('val:', val, 'err:', err); })
.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); })
.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); })
(function (err, val) { console.info('val:', val, 'err:', err); })
.then(
function (val) { console.info('val:', val); },
function (err) { console.error('err:', err); });
convert()
converts standard promise to promise-thunk.
p
: standard promise or thenable object.promisify()
converts node style function into a function returns promise-thunk.
you can use fs.exists()
and child_process.exec()
also.
ctx
: context object. default: this or undefined.fn
: node-style normal function.options
: options object.
context
: context object.also thenable, yieldable, callable.
pg
example:var pg = require('pg');
var pg_connect = PromiseThunk.promisify(pg, pg.connect); // -> yield pg_connect()
var client_query = PromiseThunk.promisify(client, client.query); // -> yield client_query()
promisify()
defines method promisified function returns promise-thunk.
object
: target object.method
: method name string.options
: method name suffix or postfix. default: 'Async'. or options object.
suffix
: method name suffix or postfix. default: 'Async'.postfix
: method name suffix or postfix. default: 'Async'.pg
example:var pg = require('pg');
PromiseThunk.promisify(pg, 'connect', {suffix: 'A'}); // -> yield pg.connectA()
PromiseThunk.promisify(pg.Client.prototype, 'connect'); // -> yield client.connectAsync()
PromiseThunk.promisify(pg.Client.prototype, 'query'); // -> yield client.queryAsync()
promisifyAll()
defines all methods promisified function returns promise-thunk.
object
: target object.options
: method name suffix or postfix. default: 'Async'. or options object.
suffix
: method name suffix or postfix. default: 'Async'.postfix
: method name suffix or postfix. default: 'Async'.fs
example:var fs = require('fs');
PromiseThunk.promisifyAll(fs, {suffix: 'A'}); // -> yield fs.readFileA()
pg
example:var pg = require('pg');
PromiseThunk.promisifyAll(pg.constructor.prototype, {suffix: 'A'}); // -> yield pg.connectA()
PromiseThunk.promisifyAll(pg.Client.prototype); // -> yield client.connectAsync()
// -> yield client.queryAsync()
thunkify()
converts node style function into a thunkified function.
you can use fs.exists()
and child_process.exec()
also.
ctx
: context object. default: this or undefined.fn
: node-style normal function with callback.options
: options object.
context
: context object.also yieldable, callable.
pg
example:var pg = require('pg');
var pg_connect = thunkify(pg, pg.connect); // -> yield pg_connect()
var client_query = thunkify(client, client.query); // -> yield client_query()
thunkify()
defines method thunkified function returns thunk.
object
: target object.method
: method name string.options
: method name suffix or postfix. default: 'Async'. or options object.
suffix
: method name suffix or postfix. default: 'Async'.postfix
: method name suffix or postfix. default: 'Async'.pg
example:var pg = require('pg');
PromiseThunk.thunkify(pg, 'connect', {suffix: 'A'}); // -> yield pg.connectA()
PromiseThunk.thunkify(pg.Client.prototype, 'connect'); // -> yield client.connectAsync()
PromiseThunk.thunkify(pg.Client.prototype, 'query'); // -> yield client.queryAsync()
thunkifyAll()
defines all methods thunkified function returns thunk.
object
: target object.options
: method name suffix or postfix. default: 'Async'. or options object.
suffix
: method name suffix or postfix. default: 'Async'.postfix
: method name suffix or postfix. default: 'Async'.fs
example:var fs = require('fs');
PromiseThunk.thunkifyAll(fs, {suffix: 'A'}); // -> yield fs.readFileA()
pg
example:var pg = require('pg');
PromiseThunk.thunkifyAll(pg.constructor.prototype, {suffix: 'A'}); // -> yield pg.connectA()
PromiseThunk.thunkifyAll(pg.Client.prototype); // -> yield client.connectAsync()
// -> yield client.queryAsync()
how to use promise.
p = p.then(
function resolved(value) {},
function rejected(error) {});
example
p = p.then(
function resolved(value) {
console.info(value);
},
function rejected(error) {
console.error(error);
});
how to catch error from promise.
p = p.catch(
function rejected(error) {});
or
when you use old browser
p = p['catch'](
function rejected(error) {});
wait for all promises.
p = PromiseThunk.all([promise, ...]);
get value or error of first finished promise.
p = PromiseThunk.race([promise, ...]);
get resolved promise.
p = PromiseThunk.resolve(value or promise);
get rejected promise.
p = PromiseThunk.reject(error);
get resolved (accepted) promise.
p = PromiseThunk.accept(value);
make deferred object with promise.
dfd = PromiseThunk.defer();
// -> {promise, resolve, reject}
npm promise-light
npm aa
npm co
MIT
FAQs
Promise and thunk
The npm package promise-thunk receives a total of 138 weekly downloads. As such, promise-thunk popularity was classified as not popular.
We found that promise-thunk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.