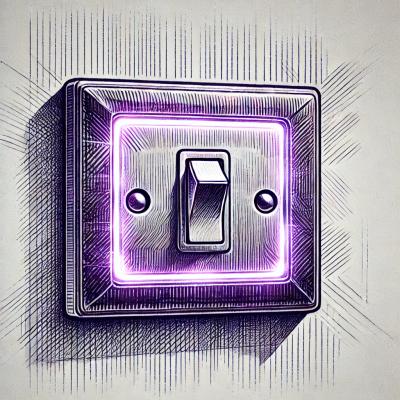
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
[](https://nodei.co/npm/rcommands/) # RCommands A powerfull command handler for [discord.js](https://discord.js.org) bots
A powerfull command handler for discord.js bots
NPM
npm install rcommands
After you successfully installed RCommands, you need to implement it to your bot. To do so:
const { Client } = require("discord.js")
const RCommands = require("rcommands")
const client = new Client()
const db = {
dbOptions: {
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
keepAlive: true,
},
mongoPath: "mongodb://..." // your mongodb connection uri
}
client.on("ready", async () => {
const rcommands = await new RCommands(client, { // initialize a new RCommands instance
cmdsDir: `${__dirname}/commands`, // your commands folder
db
})
.setDefaultPrefix("!")
rcommands.cmder(rcommands)
})
client.login("your discord app token")
It is time to create our first command module!
Create a folder into the "commands" directory named "test" then, create a file named module.js inside it, then add the following contents to it.
module.exports = {
key: "test",
name: "Test",
description: "Testing commands"
}
Now its time to create a simple ping command
// file name: ping.js
// folder: ./commands/test
module.exports = {
name: "ping", // the command name
module: "test" // the command module
aliases: ["p"], // optional
execute: (client, message, args, rClient) => {
message.channel.send(`${client.ws.ping}ms!`)
}
}
FAQs
[](https://nodei.co/npm/rcommands/) # RCommands A powerfull command handler for [discord.js](https://discord.js.org) bots
The npm package rcommands receives a total of 6 weekly downloads. As such, rcommands popularity was classified as not popular.
We found that rcommands demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.