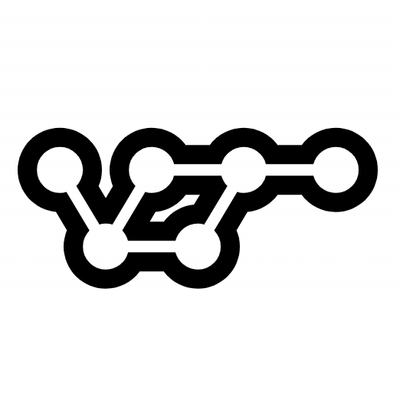
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-appsflyer
Advanced tools
This React Natie Library uses the AppsFlyer 4.6.0 library for both iOS and Android
$ npm install react-native-appsflyer --save
appsFlyerFramework
to podfile
and run pod install
.Example:
use_frameworks!
target 'demo' do
pod 'AppsFlyerFramework'
end
Don't use CocoaPods? please see their DOCS .
Create bridge between your application and appsFlyerFramework
:
In XCode ➜ project navigator, right click Libraries
➜ Add Files to [your project's name]
Go to node_modules
➜ react-native-appsflyer
and add RNAppsFlyer.xcodeproj
Build your project, It will generate libRNAppsFlyer.a
file:
Run your project (Cmd+R
) or through CLI run: react-native run-ios
dependencies {
...
compile project(':react-native-appsflyer')
}
include ':react-native-appsflyer'
project(':react-native-appsflyer').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-appsflyer/android')
Build project so you should get following dependency (see an Image):
Add:
import com.appsflyer.reactnative.RNAppsFlyerPackage;
In the getPackages()
method register the module:
new RNAppsFlyerPackage(MainApplication.this)
So getPackages()
should look like:
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new RNAppsFlyerPackage(MainApplication.this),
//.....
);
}
##API Methods {#api-methods}
Call module by adding:
import appsFlyer from 'react-native-appsflyer';
#####appsFlyer.initSdk(options, callback): void
{#init-sdk}
initializes the SDK.
parameter | type | description |
---|---|---|
options | Object | SDK configuration |
options
name | type | default | description |
---|---|---|---|
devKey | string | Appsflyer Dev key | |
appId | string | Apple Application ID (for iOS only) | |
isDebug | boolean | false | debug mode (optional) |
Example:
let options = {
devKey: 'WdpTVAcYwmxsaQ4WeTspmh',
appId: "975313579",
isDebug: true
};
appsFlyer.initSdk(options, (error, result) => {
if (error) {
console.error(error);
} else {
//..
}
})
#####appsFlyer.trackEvent(eventName, eventValues, callback): void
{#trackEvent}
trackEvent
method allows you to send in-app events to AppsFlyer analytics. This method allows you to add events dynamically by adding them directly to the application code.parameter | type | description |
---|---|---|
eventName | String | custom event name, is presented in your dashboard. See the Event list HERE |
eventValue | Object | event details |
Example:
const eventName = "af_add_to_cart";
const eventValues = {
"af_content_id": "id123",
"af_currency":"USD",
"af_revenue": "2"
};
appsFlyer.trackEvent(eventName, eventValues, (error, result) => {
if (error) {
console.error(error);
} else {
//...
}
})
#####appsFlyer.onInstallConversionData(callback): function:unregister
{#onInstallConversionData}
Accessing AppsFlyer Attribution / Conversion Data from the SDK (Deferred Deeplinking). Read more: Android, iOS
parameter | type | description |
---|---|---|
callback | function | returns object |
status
: "success"
or "failure"
if SDK returned error on onInstallConversionData
event handlertype
:"onAppOpenAttribution"
- returns deep linking data (non-organic)"onInstallConversionDataLoaded"
- called on each launch"onAttributionFailure"
"onInstallConversionFailure"
data
: some metadata,
for example:{
"status": "success",
"type": "onInstallConversionDataLoaded",
"data": {
"af_status": "Organic",
"af_message": "organic install"
}
}
Example:
this.onInstallConversionDataCanceller = appsFlyer.onInstallConversionData(
(data) => {
console.log(data);
}
);
The appsFlyer.onInstallConversionData
returns function to unregister this event listener. Actually it calls NativeAppEventEmitter.remove()
Example:
componentWillUnmount() {
if(this.onInstallConversionDataCanceller){
this.onInstallConversionDataCanceller();
}
}
#####appsFlyer.getAppsFlyerUID(callback): void
{#getAppsFlyerUID}
Get AppsFlyer’s proprietary Device ID. The AppsFlyer Device ID is the main ID used by AppsFlyer in Reports and APIs.
Example:
appsFlyer.getAppsFlyerUID((error, appsFlyerUID) => {
if (error) {
console.error(error);
} else {
console.log("on getAppsFlyerUID: " + appsFlyerUID);
}
})
#####appsFlyer.trackLocation(longitude, latitude, callback(error, coords): void
(iOS only) {#trackLocation}
Get AppsFlyer’s proprietary Device ID. The AppsFlyer Device ID is the main ID used by AppsFlyer in Reports and APIs.
parameter | type | description |
---|---|---|
error | String | Error callback - called on getAppsFlyerUID failure |
appsFlyerUID | string | The AppsFlyer Device ID |
Example:
const latitude = -18.406655;
const longitude = 46.406250;
appsFlyer.trackLocation(longitude, latitude, (error, coords) => {
if (error) {
console.error(error);
} else {
this.setState( { ...this.state, trackLocation: coords });
}
})
})
##Demo
This plugin has a demo
project bundled with it. To give it a try , clone this repo and from root a.e. react-native-appsflyer
execute the following:
npm run setup
npm run demo.ios
or npm run demo.ios
will run for the appropriate platform.npm run ios-pod
to run Podfile
under demo
projectFAQs
React Native Appsflyer plugin
The npm package react-native-appsflyer receives a total of 53,448 weekly downloads. As such, react-native-appsflyer popularity was classified as popular.
We found that react-native-appsflyer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.