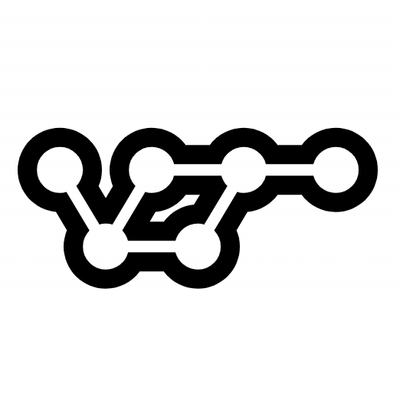
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-compressor
Advanced tools
react-native-compressor package is a set of functions that allow you compress Image
,Audio
and Video
Using Yarn
yarn add react-native-compressor
Using Npm
npm install react-native-compressor --save
Automatic linking is supported for both Android
and IOS
Note: If you are using react-native version 0.60 or higher you don't need to link this package.
react-native link react-native-compressor
Libraries
➜ Add Files to [your project's name]
node_modules
➜ react-native-compressor
and add Compressor.xcodeproj
libCompressor.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)<android/app/src/main/java/[...]/MainActivity.java
import com.reactnativecompressor.CompressorPackage;
to the imports at the top of the filenew CompressorPackage()
to the list returned by the getPackages()
methodandroid/settings.gradle
:
include ':react-native-compressor'
project(':react-native-compressor').projectDir = new File(rootProject.projectDir,'../node_modules/react-native-compressor/android')
android/app/build.gradle
:
compile project(':react-native-compressor')
import { Image } from 'react-native-compressor';
const result = await Image.compress('file://path_of_file/image.jpg', {
compressionMethod: 'auto',
});
Here is this package comparison of images compression with WhatsApp
import { Image } from 'react-native-compressor';
const result = await Image.compress('file://path_of_file/image.jpg', {
maxWidth: 1000,
quality: 0.8,
});
import { Audio } from 'react-native-compressor';
const result = await Audio.compress(
'file://path_of_file/file_example_MP3_2MG.mp3',
{ quality: 'medium' }
);
import { Video } from 'react-native-compressor';
const result = await Video.compress(
'file://path_of_file/BigBuckBunny.mp4',
{
compressionMethod: 'auto',
},
(progress) => {
if (backgroundMode) {
console.log('Compression Progress: ', progress);
} else {
setCompressingProgress(progress);
}
}
);
Here is this package comparison of video compression with WhatsApp
import { Video } from 'react-native-compressor';
const result = await Video.compress(
'file://path_of_file/BigBuckBunny.mp4',
{},
(progress) => {
if (backgroundMode) {
console.log('Compression Progress: ', progress);
} else {
setCompressingProgress(progress);
}
}
);
compress(value: string, options?: CompressorOptions): Promise<string>
Compresses the input file URI or base-64 string with the specified options. Promise returns a string after compression has completed. Resizing will always keep the original aspect ratio of the image, the maxWidth
and maxHeight
are used as a boundary.
compressionMethod: compressionMethod
(default: "manual")if you want to compress images like whatsapp then make this prop auto
. Can be either manual
or auto
, defines the Compression Method.
maxWidth: number
(default: 1280)The maximum width boundary used as the main boundary in resizing a landscape image.
maxHeight: number
(default: 1280)The maximum height boundary used as the main boundary in resizing a portrait image.
quality: number
(default: 0.8)The quality modifier for the JPEG
file format, can be specified when output is PNG
but will be ignored.
input: InputType
(default: uri)Can be either uri
or base64
, defines the contentents of the value
parameter.
output: OutputType
(default: jpg)Can be either jpg
or png
, defines the output image format.
returnableOutputType: ReturnableOutputType
(default: uri)Can be either uri
or base64
, defines the Returnable output image format.
compress(url: string, options?: audioCompresssionType): Promise<string>
quality: qualityType
(default: medium)low
| medium
| high
compress(url: string, options?: videoCompresssionType , onProgress?: (progress: number)): Promise<string>
compressionMethod: compressionMethod
(default: "manual")if you want to compress videos like whatsapp then make this prop auto
. Can be either manual
or auto
, defines the Compression Method.
maxSize: number
(default: 640)The maximum size can be height in case of portrait video or can be width in case of landscape video.
bitrate: string
bitrate of video which reduce or increase video size. if compressionMethod will auto then this prop will not work
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
FAQs
Compress Image, Video, and Audio same like Whatsapp & Auto/Manual Compression | Background Upload | Download File | Create Video Thumbnail
The npm package react-native-compressor receives a total of 44,797 weekly downloads. As such, react-native-compressor popularity was classified as popular.
We found that react-native-compressor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.