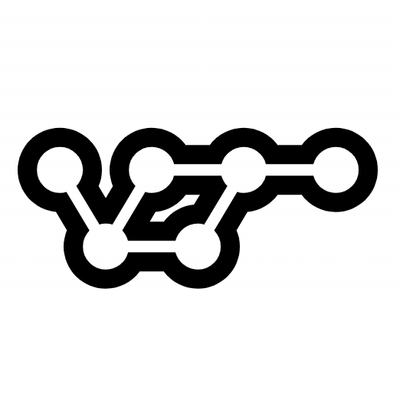
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-flip-component
Advanced tools
npm install react-native-flip-component --save
Pass a boolean, front component, and back component as props. The boolean will determine if the front or back component should be displayed.
GIF coming soon...
Name | Required? | Description |
---|---|---|
isFlipped | true | boolean |
frontView | true | Component |
backView | true | Component |
scale | false | defaults to 0.8 |
scaleDuration | false | defaults to 100 |
frontPerspective | false | defaults to 1000 |
backPerspective | false | defaults to 1000 |
rotateDuration | false | defaults to 300 |
containerStyles | false | defaults to { flex: 1 } |
frontStyles | false | defaults to null |
backStyles | false | defaults to null |
import React, { Component } from 'react';
import FlipComponent from 'react-native-flip-component';
import { View, Button, Text } from 'react-native';
class Example extends Component {
constructor(props) {
super(props);
this.state = {
isFlipped: false;
};
}
render() {
<View>
<FlipComponent
isFlipped={this.state.isFlipped}
frontView={
<View>
<Text style={{ textAlign: 'center' }}>Front Side</Text>
</View>
}
backView={
<View>
<Text style={{ textAlign: 'center' }}>Back Side</Text>
</View>
}
/>
<Button
onPress={() => this.setState({ isFlipped: !this.state.isFlipped })}
title="Flip"
/>
</View>
}
}
FAQs
Double-sided React component that flips 180 degrees
We found that react-native-flip-component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.