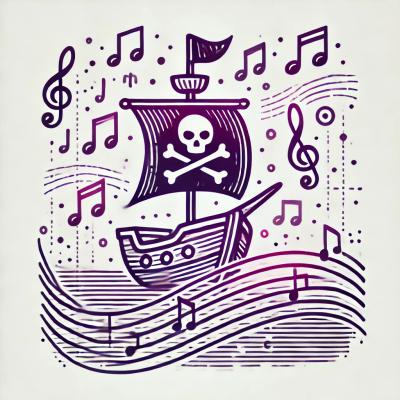
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
react-native-google-places-autocomplete
Advanced tools
Customizable Google Places autocomplete component for iOS and Android React-Native apps
The react-native-google-places-autocomplete package is a customizable React Native component that provides a Google Places autocomplete input field. It allows users to search for places and get location details using the Google Places API.
Autocomplete Input
This feature provides an input field where users can type in a location name, and it will suggest places using Google Places API. The code sample demonstrates how to set up the autocomplete input and handle the selection of a place.
import React from 'react';
import { GooglePlacesAutocomplete } from 'react-native-google-places-autocomplete';
const GooglePlacesInput = () => {
return (
<GooglePlacesAutocomplete
placeholder="Search"
onPress={(data, details = null) => {
console.log(data, details);
}}
query={{
key: 'YOUR_API_KEY',
language: 'en',
}}
/>
);
};
export default GooglePlacesInput;
Customizable Components
This feature allows customization of the autocomplete component, including styles and behavior. The code sample shows how to customize the input field, such as setting the minimum length for search, autofocus, and styling the input container.
import React from 'react';
import { GooglePlacesAutocomplete } from 'react-native-google-places-autocomplete';
const CustomGooglePlacesInput = () => {
return (
<GooglePlacesAutocomplete
placeholder="Search"
minLength={2}
autoFocus={false}
returnKeyType={'search'}
listViewDisplayed={false}
fetchDetails={true}
renderDescription={row => row.description}
onPress={(data, details = null) => {
console.log(data, details);
}}
query={{
key: 'YOUR_API_KEY',
language: 'en',
}}
styles={{
textInputContainer: {
width: '100%',
},
description: {
fontWeight: 'bold',
},
predefinedPlacesDescription: {
color: '#1faadb',
},
}}
/>
);
};
export default CustomGooglePlacesInput;
react-native-maps provides map components for React Native applications, allowing for the integration of maps and location-based features. While it does not offer autocomplete functionality, it can be used in conjunction with react-native-google-places-autocomplete to display selected locations on a map.
react-native-geolocation-service is a package that provides high accuracy geolocation features for React Native apps. It focuses on obtaining the device's current location rather than providing place autocomplete functionality, making it complementary to react-native-google-places-autocomplete.
react-native-google-places is another package that provides Google Places API integration for React Native. It offers similar functionalities to react-native-google-places-autocomplete, such as place search and details retrieval, but with a different API and potentially less customization options.
Customizable Google Places autocomplete component for iOS and Android React-Native apps
npm install react-native-google-places-autocomplete --save
import React from 'react';
import { View, Image } from 'react-native';
import { GooglePlacesAutocomplete } from 'react-native-google-places-autocomplete';
const homePlace = { description: 'Home', geometry: { location: { lat: 48.8152937, lng: 2.4597668 } }};
const workPlace = { description: 'Work', geometry: { location: { lat: 48.8496818, lng: 2.2940881 } }};
const GooglePlacesInput = () => {
return (
<GooglePlacesAutocomplete
placeholder='Search'
minLength={2} // minimum length of text to search
autoFocus={false}
returnKeyType={'search'} // Can be left out for default return key https://facebook.github.io/react-native/docs/textinput.html#returnkeytype
listViewDisplayed='auto' // true/false/undefined
fetchDetails={true}
renderDescription={row => row.description} // custom description render
onPress={(data, details = null) => { // 'details' is provided when fetchDetails = true
console.log(data, details);
}}
getDefaultValue={() => ''}
query={{
// available options: https://developers.google.com/places/web-service/autocomplete
key: 'YOUR API KEY',
language: 'en', // language of the results
types: '(cities)' // default: 'geocode'
}}
styles={{
textInputContainer: {
width: '100%'
},
description: {
fontWeight: 'bold'
},
predefinedPlacesDescription: {
color: '#1faadb'
}
}}
currentLocation={true} // Will add a 'Current location' button at the top of the predefined places list
currentLocationLabel="Current location"
nearbyPlacesAPI='GooglePlacesSearch' // Which API to use: GoogleReverseGeocoding or GooglePlacesSearch
GoogleReverseGeocodingQuery={{
// available options for GoogleReverseGeocoding API : https://developers.google.com/maps/documentation/geocoding/intro
}}
GooglePlacesSearchQuery={{
// available options for GooglePlacesSearch API : https://developers.google.com/places/web-service/search
rankby: 'distance',
types: 'food'
}}
filterReverseGeocodingByTypes={['locality', 'administrative_area_level_3']} // filter the reverse geocoding results by types - ['locality', 'administrative_area_level_3'] if you want to display only cities
predefinedPlaces={[homePlace, workPlace]}
debounce={200} // debounce the requests in ms. Set to 0 to remove debounce. By default 0ms.
renderLeftButton={() => <Image source={require('path/custom/left-icon')} />}
renderRightButton={() => <Text>Custom text after the input</Text>}
/>
);
}
GooglePlacesAutocomplete
can be easily customized to meet styles of your app. Pass styles props to GooglePlacesAutocomplete
with style object for different elements (keys for style object are listed below)
key | type |
---|---|
container | object (View) |
description | object (Text style) |
textInputContainer | object (View style) |
textInput | object (style) |
loader | object (View style) |
listView | object (ListView style) |
predefinedPlacesDescription | object (Text style) |
poweredContainer | object (View style) |
powered | object (Image style) |
separator | object (View style) |
<GooglePlacesAutocomplete
placeholder='Enter Location'
minLength={2}
autoFocus={false}
returnKeyType={'default'}
fetchDetails={true}
styles={{
textInputContainer: {
backgroundColor: 'rgba(0,0,0,0)',
borderTopWidth: 0,
borderBottomWidth:0
},
textInput: {
marginLeft: 0,
marginRight: 0,
height: 38,
color: '#5d5d5d',
fontSize: 16
},
predefinedPlacesDescription: {
color: '#1faadb'
},
}}
currentLocation={false}
/>
styles
parameterkey prop
warning and added loading indicator.componentDidMount()
when you
already have the default value set.react-native
peerDependecy. (> 0.46)ListView
to Flatlist
.onPress
event.debounce
to 0
. Fixed debounce typing lag.isRowScrollable
prop.underlineColorAndroid
, listUnderlayColor
, renderLeftButton
, renderRightButton
props. Added nearbyPlacesAPI
option None
.FAQs
Customizable Google Places autocomplete component for iOS and Android React-Native apps
The npm package react-native-google-places-autocomplete receives a total of 111,298 weekly downloads. As such, react-native-google-places-autocomplete popularity was classified as popular.
We found that react-native-google-places-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.