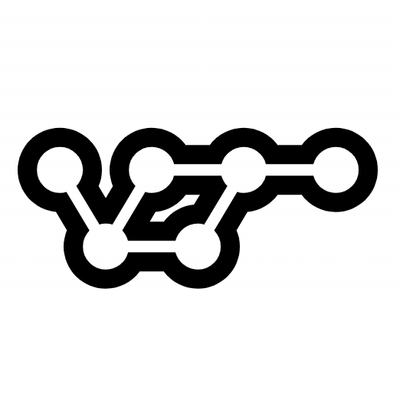
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-native-webrtc
Advanced tools
A WebRTC module for React Native.
Currently support for iOS only.
Support video and audio communication. Not support DataChannel now.
You can use it to build an app that can communicate with web browser.
The iOS Library is based on libWebRTC.a(It's build by webrtc-build-scripts and you can download it here)
1.) Run react-native init RCTWebRTCDemo
to create your react native project. (RCTWebRTCDemo can be any name you like)
2.) npm install react-native-webrtc --save
3.) Open RCTWebRTCDemo/node_modules/react-native-webrtc
4.) Drag RCTWebRTC
directory to you project. Uncheck Copy items if needed
and select Added folders: Create groups
5.) Select your target, select Build Phases
, open Link Binary With Libraries
, add these libraries
AVFoundation.framework
AudioToolbox.framework
CoreGraphics.framework
GLKit.framework
CoreAudio.framework
CoreVideo.framework
VideoToolbox.framework
libc.tbd
libsqlite3.tbd
libstdc++.tbd
6.) select Build Settings
, find Library Search Paths
, add $(SRCROOT)/../node_modules/react-native-webrtc
with recursive
7.) Maybe you have to set Dead Code Stripping
to No
and Enable Bitcode
to No
.
Now, you can use WebRTC like in browser. In your index.ios.js, you can require WebRTC to import RTCPeerConnection, RTCSessionDescription, etc.
var WebRTC = require('react-native-webrtc');
var {
RTCPeerConnection,
RTCMediaStream,
RTCIceCandidate,
RTCSessionDescription,
RTCView
} = WebRTC;
Anything about using RTCPeerConnection, RTCSessionDescription and RTCIceCandidate is like browser. However, render video stream should be used by React way.
Rendering RTCView.
var container;
var RCTWebRTCDemo = React.createClass({
getInitialState: function() {
return {videoSrc: null};
},
componentDidMount: function() {
container = this;
},
render: function() {
return (
<View>
<RTCView src={this.state.videoSrc}/>
</View>
);
}
});
And set stream to RTCView
container.setState({videoSrc: stream.objectId});
The demo project is https://github.com/oney/RCTWebRTCDemo
And you will need a signaling server. I have written a signaling server http://react-native-webrtc.herokuapp.com/ (the repository is https://github.com/oney/react-native-webrtc-server).
You can open this website in browser, and then set it as signaling server in the app, and run the app. After you enter the same room ID, the video stream will be connected.
FAQs
WebRTC for React Native
The npm package react-native-webrtc receives a total of 20,014 weekly downloads. As such, react-native-webrtc popularity was classified as popular.
We found that react-native-webrtc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.