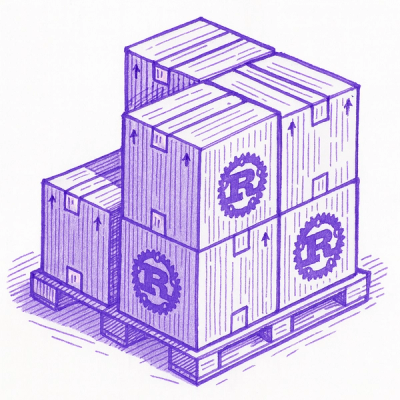
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
react-next-theme
Advanced tools
npm react-next-theme is a simple and flexible theme switcher for React and Next.js. It enables seamless toggling between light and dark modes, supports user preferences, and stores the selected theme in local storage. Easily integrate it into any Next.js
Here's an updated README.md file with the new package name, react-next-theme
, and ready for users to copy-paste the code snippets and installation instructions:
# react-next-theme
`react-next-theme` is a simple and flexible theme switcher for React and Next.js. It allows for seamless toggling between light and dark modes, supports user preferences, and stores the selected theme in local storage.
## Features
- **Automatic Theme Detection**: Detects system-level theme preferences (light/dark).
- **Persistent Theme**: Automatically saves and loads the user's preferred theme using `localStorage`.
- **Easy Integration**: Simple integration with React and Next.js projects.
- **CSS Variables**: Uses CSS variables for dynamic theming.
## Installation
You can install the `react-next-theme` package using npm or yarn:
```bash
npm install react-next-theme
or
yarn add react-next-theme
To use the package in a React application, follow these steps:
Wrap Your App with ThemeProvider
In your main file (e.g., src/main.jsx
or src/index.js
), wrap your app in the ThemeProvider
to provide theme context to the entire application.
// src/main.jsx
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import './index.css'; // Import your global CSS
import { ThemeProvider } from 'react-next-theme';
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<ThemeProvider>
<App />
</ThemeProvider>
</React.StrictMode>
);
Use useTheme
to Toggle Theme
In your components, you can use the useTheme
hook to toggle between light and dark modes:
// src/App.jsx
import React from 'react';
import { useTheme } from 'react-next-theme';
const App = () => {
const { theme, toggleTheme } = useTheme();
return (
<div>
<h1>Current Theme: {theme}</h1>
<button onClick={toggleTheme}>
Toggle to {theme === 'light' ? 'Dark' : 'Light'} Mode
</button>
</div>
);
};
export default App;
Add CSS for Themes
Add CSS for your light and dark themes in your global styles (src/index.css
):
/* src/index.css */
/* Default Light Theme */
:root {
--bg-color: #ffffff;
--text-color: #213547;
--link-color: #646cff;
--link-hover-color: #747bff;
--button-bg-color: #f9f9f9;
--button-text-color: #213547;
}
body {
background-color: var(--bg-color);
color: var(--text-color);
}
[data-theme='dark'] {
--bg-color: #242424;
--text-color: rgba(255, 255, 255, 0.87);
--link-color: #646cff;
--link-hover-color: #535bf2;
--button-bg-color: #1a1a1a;
--button-text-color: #ffffff;
}
react-next-theme
works seamlessly with both the App Router and Pages Router in Next.js.
Wrap Your App with ThemeProvider
In app/layout.js
or app/layout.tsx
, wrap your app with ThemeProvider
:
// app/layout.js
import './globals.css'; // Import global CSS
import { ThemeProvider } from 'react-next-theme';
export default function RootLayout({ children }) {
return (
<html lang="en">
<body>
<ThemeProvider>{children}</ThemeProvider>
</body>
</html>
);
}
Use useTheme
to Toggle Theme
In any page or component, use the useTheme
hook:
// app/page.js
import { useTheme } from 'react-next-theme';
export default function HomePage() {
const { theme, toggleTheme } = useTheme();
return (
<div>
<h1>Current Theme: {theme}</h1>
<button onClick={toggleTheme}>
Toggle to {theme === 'light' ? 'Dark' : 'Light'} Mode
</button>
</div>
);
}
Add Global CSS
Add your CSS for light and dark themes in globals.css
:
/* styles/globals.css */
:root {
--bg-color: #ffffff;
--text-color: #213547;
--link-color: #646cff;
--link-hover-color: #747bff;
--button-bg-color: #f9f9f9;
--button-text-color: #213547;
}
body {
background-color: var(--bg-color);
color: var(--text-color);
}
[data-theme='dark'] {
--bg-color: #242424;
--text-color: rgba(255, 255, 255, 0.87);
--link-color: #646cff;
--link-hover-color: #535bf2;
--button-bg-color: #1a1a1a;
--button-text-color: #ffffff;
}
Wrap Your App with ThemeProvider
In pages/_app.js
, wrap your app with ThemeProvider
:
// pages/_app.js
import '../styles/globals.css';
import { ThemeProvider } from 'react-next-theme';
export default function MyApp({ Component, pageProps }) {
return (
<ThemeProvider>
<Component {...pageProps} />
</ThemeProvider>
);
}
Use useTheme
to Toggle Theme
In any page or component, use the useTheme
hook:
// pages/index.js
import { useTheme } from 'react-next-theme';
export default function Home() {
const { theme, toggleTheme } = useTheme();
return (
<div>
<h1>Current Theme: {theme}</h1>
<button onClick={toggleTheme}>
Toggle to {theme === 'light' ? 'Dark' : 'Light'} Mode
</button>
</div>
);
}
Add Global CSS
Add your CSS in globals.css
:
/* styles/globals.css */
:root {
--bg-color: #ffffff;
--text-color: #213547;
--link-color: #646cff;
--link-hover-color: #747bff;
--button-bg-color: #f9f9f9;
--button-text-color: #213547;
}
body {
background-color: var(--bg-color);
color: var(--text-color);
}
[data-theme='dark'] {
--bg-color: #242424;
--text-color: rgba(255, 255, 255, 0.87);
--link-color: #646cff;
--link-hover-color: #535bf2;
--button-bg-color: #1a1a1a;
--button-text-color: #ffffff;
}
The react-next-theme
package automatically stores the user's theme preference in localStorage
and loads it on page reload. This ensures a consistent experience across sessions.
react-next-theme
also detects the user's system-level theme preference (light or dark) using the prefers-color-scheme
media query and applies it as the default theme if no preference is stored in localStorage
.
react-next-theme
is licensed under the MIT License.
FAQs
A simple and flexible theme switcher for React and Next.js, supporting light and dark modes with localStorage and system preferences.
The npm package react-next-theme receives a total of 9 weekly downloads. As such, react-next-theme popularity was classified as not popular.
We found that react-next-theme demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.