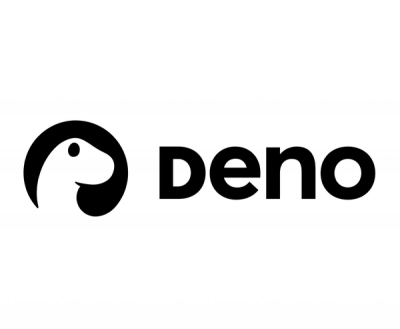
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
react-next-theme
Advanced tools
A simple and flexible theme switcher for React and Next.js, supporting light and dark modes with localStorage and system preferences.
Sure! Here's the complete README.md
file, including instructions for both npm and yarn, detailed usage for React, Next.js (Pages Router and App Router), and the CSS you provided.
react-next-theme
is a simple and flexible theme switcher for React and Next.js applications, supporting light and dark modes. It provides a seamless solution for managing themes across your applications with automatic theme detection based on system preferences and local storage.
You can install the package via npm or yarn.
npm install react-next-theme
yarn add react-next-theme
To ensure the theme is correctly applied to your application, include the following CSS in your global styles (index.css
or globals.css
depending on your setup):
you can change color as you want.
:root {
--background-color: white;
--text-color: black;
}
html[data-theme='dark'] {
--background-color: black;
--text-color: white;
}
body {
background-color: var(--background-color);
color: var(--text-color);
transition: background-color 0.3s, color 0.3s;
}
This CSS sets the background and text colors based on the data-theme
attribute applied to the <html>
element.
To prevent a white flash on page load, you need to inject a script that applies the correct theme before React mounts. Modify your public/index.html
or index.html
file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>React App</title>
<!-- Insert the theme initialization style of the avobe style -->
<!-- <link rel="stylesheet" href="insert css file link"> -->
<!-- Insert the theme initialization script -->
<script>
(function() {
const storedTheme = localStorage.getItem('theme');
const prefersDark = window.matchMedia('(prefers-color-scheme: dark)').matches;
const theme = storedTheme || (prefersDark ? 'dark' : 'light');
document.documentElement.setAttribute('data-theme', theme);
})();
</script>
</head>
<body>
<div id="root"></div>
</body>
</html>
ThemeProvider
in src/index.js
or src/main.js
Wrap your app with the ThemeProvider
to handle dynamic theme switching:
// src/index.js or src/main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { ThemeProvider } from 'react-next-theme';
ReactDOM.render(
<ThemeProvider>
<App />
</ThemeProvider>,
document.getElementById('root')
);
useTheme
Hook in Your Componentsyou can change the button or modify as your need
// src/App.js
import React from 'react';
import { useTheme } from 'react-next-theme';
function App() {
const { theme, toggleTheme } = useTheme();
return (
<div>
<h1>{theme === 'light' ? 'Light Mode' : 'Dark Mode'}</h1>
<button onClick={toggleTheme}>
{theme === 'light' ? 'Switch to Dark Mode' : 'Switch to Light Mode'}
</button>
</div>
);
}
export default App;
_document.js
To prevent the white flash, you need to inject the theme initialization script using Next.js' _document.js
file:
// pages/_document.js
import { Html, Head, Main, NextScript } from 'next/document';
import { getThemeScript } from 'react-next-theme';
export default function Document() {
return (
<Html>
<Head>
{/* Inject the theme initialization script */}
<script dangerouslySetInnerHTML={{ __html: getThemeScript() }} />
</Head>
<body>
<Main />
<NextScript />
</body>
</Html>
);
}
ThemeProvider
in _app.js
// pages/_app.js
import { ThemeProvider } from 'react-next-theme';
function MyApp({ Component, pageProps }) {
return (
<ThemeProvider>
<Component {...pageProps} />
</ThemeProvider>
);
}
export default MyApp;
useTheme
Hook in Components// components/Header.js
import { useTheme } from 'react-next-theme';
function Header() {
const { theme, toggleTheme } = useTheme();
return (
<header>
<button onClick={toggleTheme}>
{theme === 'light' ? 'Switch to Dark Mode' : 'Switch to Light Mode'}
</button>
</header>
);
}
export default Header;
layout.js
For Next.js 13 (App Router), modify the app/layout.js
file to inject the theme initialization script:
// app/layout.js
import { getThemeScript } from 'react-next-theme';
export const metadata = {
title: 'My App',
};
export default function RootLayout({ children }) {
return (
<html lang="en">
<head>
{/* Inject the theme initialization script */}
<script dangerouslySetInnerHTML={{ __html: getThemeScript() }} />
</head>
<body>
{children}
</body>
</html>
);
}
ThemeProvider
In Next.js (App Router), wrap your entire app with the ThemeProvider
:
// app/layout.js
import { ThemeProvider } from 'react-next-theme';
export default function RootLayout({ children }) {
return (
<ThemeProvider>
{children}
</ThemeProvider>
);
}
useTheme
Hook in Components// components/Header.js
import { useTheme } from 'react-next-theme';
function Header() {
const { theme, toggleTheme } = useTheme();
return (
<header>
<button onClick={toggleTheme}>
{theme === 'light' ? 'Switch to Dark Mode' : 'Switch to Light Mode'}
</button>
</header>
);
}
export default Header;
If you prefer to use an external script file, you can place the theme initialization script inside the public/
folder and reference it using the <Script>
component in Next.js:
public/react-next-theme-script.js
:// public/react-next-theme-script.js
(function() {
const storedTheme = localStorage.getItem('theme');
const prefersDark = window.matchMedia('(prefers-color-scheme: dark)').matches;
const theme = storedTheme || (prefersDark ? 'dark' : 'light');
document.documentElement.setAttribute('data-theme', theme);
})();
_document.js
or layout.js
import Script from 'next/script';
<Script src="/react-next-theme-script.js" strategy="beforeInteractive" />
This will load the theme initialization script from the public/
directory.
MIT License
Feel free to modify or contribute to the project. Happy coding!
FAQs
A simple and flexible theme switcher for React and Next.js, supporting light and dark modes with localStorage and system preferences.
The npm package react-next-theme receives a total of 1 weekly downloads. As such, react-next-theme popularity was classified as not popular.
We found that react-next-theme demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.