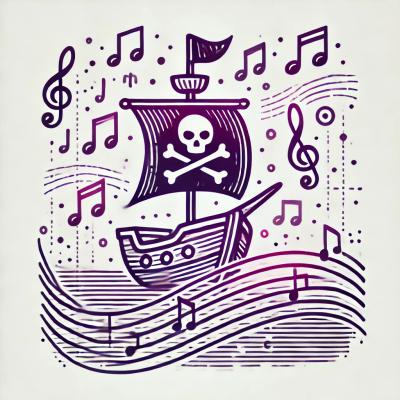
Research
Security News
Malicious PyPI Package Exploits Deezer API for Coordinated Music Piracy
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
react-responsive
Advanced tools
The react-responsive package is a utility for managing media queries in React applications. It allows developers to create responsive components that adapt to different screen sizes and orientations.
Media Query Component
This feature allows you to use media queries directly within your React components. The useMediaQuery hook can be used to conditionally render content based on the screen size, orientation, and resolution.
import { useMediaQuery } from 'react-responsive';
const Example = () => {
const isDesktopOrLaptop = useMediaQuery({ minWidth: 1224 });
const isBigScreen = useMediaQuery({ minWidth: 1824 });
const isTabletOrMobile = useMediaQuery({ maxWidth: 1224 });
const isPortrait = useMediaQuery({ orientation: 'portrait' });
const isRetina = useMediaQuery({ minResolution: '2dppx' });
return (
<div>
{isDesktopOrLaptop && <p>You are a desktop or laptop</p>}
{isBigScreen && <p>You have a huge screen</p>}
{isTabletOrMobile && <p>You are a tablet or mobile phone</p>}
{isPortrait && <p>You are in portrait orientation</p>}
{isRetina && <p>You are retina</p>}
</div>
);
};
Media Query Component with Children
This feature allows you to use the MediaQuery component to wrap children elements that should only be rendered if the media query matches. This is useful for conditionally rendering parts of your component tree based on media queries.
import { MediaQuery } from 'react-responsive';
const Example = () => (
<div>
<MediaQuery minWidth={1224}>
<p>You are a desktop or laptop</p>
</MediaQuery>
<MediaQuery minWidth={1824}>
<p>You have a huge screen</p>
</MediaQuery>
<MediaQuery maxWidth={1224}>
<p>You are a tablet or mobile phone</p>
</MediaQuery>
<MediaQuery orientation="portrait">
<p>You are in portrait orientation</p>
</MediaQuery>
<MediaQuery minResolution="2dppx">
<p>You are retina</p>
</MediaQuery>
</div>
);
react-media is a similar package that provides a declarative way to use media queries in React. It offers a Media component that can be used to conditionally render content based on media queries. Compared to react-responsive, react-media is more focused on providing a simple and declarative API for media queries.
react-responsive-mixin is a mixin for React components that allows them to respond to media query changes. It is less commonly used in modern React applications due to the shift away from mixins in favor of hooks and higher-order components. However, it can still be useful for legacy codebases that rely on mixins.
react-socks is another package for handling media queries in React. It provides a set of components and hooks for responsive design. Compared to react-responsive, react-socks offers a more modern API with hooks and context, making it a good alternative for developers looking for a more contemporary solution.
Package | react-responsive |
Description | Media queries in react for responsive design |
Browser Version | >= IE6* |
Demo |
The best supported, easiest to use react media query module.
$ npm install react-responsive --save
Hooks is a new feature available in 8.0.0!
import React from 'react'
import { useMediaQuery } from 'react-responsive'
const Example = () => {
const isDesktopOrLaptop = useMediaQuery({
query: '(min-width: 1224px)'
})
const isBigScreen = useMediaQuery({ query: '(min-width: 1824px)' })
const isTabletOrMobile = useMediaQuery({ query: '(max-width: 1224px)' })
const isPortrait = useMediaQuery({ query: '(orientation: portrait)' })
const isRetina = useMediaQuery({ query: '(min-resolution: 2dppx)' })
return (
<div>
<h1>Device Test!</h1>
{isDesktopOrLaptop && <p>You are a desktop or laptop</p>}
{isBigScreen && <p>You have a huge screen</p>}
{isTabletOrMobile && <p>You are a tablet or mobile phone</p>}
<p>Your are in {isPortrait ? 'portrait' : 'landscape'} orientation</p>
{isRetina && <p>You are retina</p>}
</div>
)
}
import MediaQuery from 'react-responsive'
const Example = () => (
<div>
<h1>Device Test!</h1>
<MediaQuery minWidth={1224}>
<p>You are a desktop or laptop</p>
<MediaQuery minWidth={1824}>
<p>You also have a huge screen</p>
</MediaQuery>
</MediaQuery>
<MediaQuery minResolution="2dppx">
{/* You can also use a function (render prop) as a child */}
{(matches) =>
matches ? <p>You are retina</p> : <p>You are not retina</p>
}
</MediaQuery>
</div>
)
To make things more idiomatic to react, you can use camel-cased shorthands to construct media queries.
For a list of all possible shorthands and value types see https://github.com/yocontra/react-responsive/blob/master/src/mediaQuery.ts#L9.
Any numbers given as shorthand will be expanded to px (1234
will become '1234px'
).
The CSS media queries in the example above could be constructed like this:
import React from 'react'
import { useMediaQuery } from 'react-responsive'
const Example = () => {
const isDesktopOrLaptop = useMediaQuery({ minWidth: 1224 })
const isBigScreen = useMediaQuery({ minWidth: 1824 })
const isTabletOrMobile = useMediaQuery({ maxWidth: 1224 })
const isPortrait = useMediaQuery({ orientation: 'portrait' })
const isRetina = useMediaQuery({ minResolution: '2dppx' })
return <div>...</div>
}
device
propAt times you may need to render components with different device settings than what gets automatically detected. This is especially useful in a Node environment where these settings can't be detected (SSR) or for testing.
orientation
, scan
, aspectRatio
, deviceAspectRatio
,
height
, deviceHeight
, width
, deviceWidth
, color
, colorIndex
, monochrome
,
resolution
and type
type
can be one of: all
, grid
, aural
, braille
, handheld
, print
, projection
,
screen
, tty
, tv
or embossed
Note: The device
property always applies, even when it can be detected (where window.matchMedia exists).
import { useMediaQuery } from 'react-responsive'
const Example = () => {
const isDesktopOrLaptop = useMediaQuery(
{ minDeviceWidth: 1224 },
{ deviceWidth: 1600 } // `device` prop
)
return (
<div>
{isDesktopOrLaptop && (
<p>
this will always get rendered even if device is shorter than 1224px,
that's because we overrode device settings with 'deviceWidth: 1600'.
</p>
)}
</div>
)
}
You can also pass device
to every useMediaQuery
hook in the components tree through a React Context.
This should ease up server-side-rendering and testing in a Node environment, e.g:
import { Context as ResponsiveContext } from 'react-responsive'
import { renderToString } from 'react-dom/server'
import App from './App'
...
// Context is just a regular React Context component, it accepts a `value` prop to be passed to consuming components
const mobileApp = renderToString(
<ResponsiveContext.Provider value={{ width: 500 }}>
<App />
</ResponsiveContext.Provider>
)
...
If you use next.js, structure your import like this to disable server-side rendering for components that use this library:
import dynamic from 'next/dynamic'
const MediaQuery = dynamic(() => import('react-responsive'), {
ssr: false
})
import { Context as ResponsiveContext } from 'react-responsive'
import { render } from '@testing-library/react'
import ProductsListing from './ProductsListing'
describe('ProductsListing', () => {
test('matches the snapshot', () => {
const { container: mobile } = render(
<ResponsiveContext.Provider value={{ width: 300 }}>
<ProductsListing />
</ResponsiveContext.Provider>
)
expect(mobile).toMatchSnapshot()
const { container: desktop } = render(
<ResponsiveContext.Provider value={{ width: 1000 }}>
<ProductsListing />
</ResponsiveContext.Provider>
)
expect(desktop).toMatchSnapshot()
})
})
Note that if anything has a device
prop passed in it will take precedence over the one from context.
onChange
You can use the onChange
callback to specify a change handler that will be called when the media query's value changes.
import React from 'react'
import { useMediaQuery } from 'react-responsive'
const Example = () => {
const handleMediaQueryChange = (matches) => {
// matches will be true or false based on the value for the media query
}
const isDesktopOrLaptop = useMediaQuery(
{ minWidth: 1224 },
undefined,
handleMediaQueryChange
)
return <div>...</div>
}
import React from 'react'
import MediaQuery from 'react-responsive'
const Example = () => {
const handleMediaQueryChange = (matches) => {
// matches will be true or false based on the value for the media query
}
return (
<MediaQuery minWidth={1224} onChange={handleMediaQueryChange}>
...
</MediaQuery>
)
}
That's it! Now you can create your application specific breakpoints and reuse them easily. Here is an example:
import { useMediaQuery } from 'react-responsive'
const Desktop = ({ children }) => {
const isDesktop = useMediaQuery({ minWidth: 992 })
return isDesktop ? children : null
}
const Tablet = ({ children }) => {
const isTablet = useMediaQuery({ minWidth: 768, maxWidth: 991 })
return isTablet ? children : null
}
const Mobile = ({ children }) => {
const isMobile = useMediaQuery({ maxWidth: 767 })
return isMobile ? children : null
}
const Default = ({ children }) => {
const isNotMobile = useMediaQuery({ minWidth: 768 })
return isNotMobile ? children : null
}
const Example = () => (
<div>
<Desktop>Desktop or laptop</Desktop>
<Tablet>Tablet</Tablet>
<Mobile>Mobile</Mobile>
<Default>Not mobile (desktop or laptop or tablet)</Default>
</div>
)
export default Example
And if you want a combo (the DRY way):
import { useMediaQuery } from 'react-responsive'
const useDesktopMediaQuery = () =>
useMediaQuery({ query: '(min-width: 1280px)' })
const useTabletAndBelowMediaQuery = () =>
useMediaQuery({ query: '(max-width: 1279px)' })
const Desktop = ({ children }) => {
const isDesktop = useDesktopMediaQuery()
return isDesktop ? children : null
}
const TabletAndBelow = ({ children }) => {
const isTabletAndBelow = useTabletAndBelowMediaQuery()
return isTabletAndBelow ? children : null
}
Chrome | 9 |
Firefox (Gecko) | 6 |
MS Edge | All |
Internet Explorer | 10 |
Opera | 12.1 |
Safari | 5.1 |
Pretty much everything. Check out these polyfills:
FAQs
Media queries in react for responsive design
We found that react-responsive demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.
Security News
Newly introduced telemetry in devenv 1.4 sparked a backlash over privacy concerns, leading to the removal of its AI-powered feature after strong community pushback.