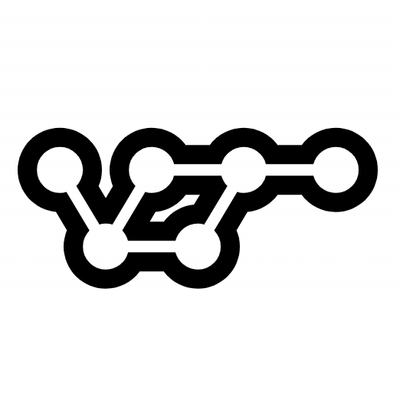
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
react-texteditor-toolkit
Advanced tools
A fully customizable and easy-to-use rich text editor built with React. Includes support for formatting, alignment, lists, and more.
Install the package via npm:
npm install your-package-name
Here's how to use the TextEditor in your project:
import React, { useState } from "react";
import TextEditor from "your-package-name";
const App = () => {
const [content, setContent] = useState("");
const handleContentChange = (e: { target: { value: string } }) => {
setContent(e.target.value);
console.log("Editor Content:", e.target.value);
};
return (
<div>
<h1>My Rich Text Editor</h1>
<TextEditor
placeholder="Start typing here..."
onChange={handleContentChange}
toolbarActions={["bold", "italic", "underline", "unorderedList", "clear"]}
contentStyle={{ border: "1px solid #ddd", padding: "10px" }}
/>
<p>Output:</p>
<div dangerouslySetInnerHTML={{ __html: content }} />
</div>
);
};
export default App;
You can customize the editor's appearance using the contentStyle, iconStyle, and headerStyle props.
<TextEditor
contentStyle={{ border: "2px solid #000", minHeight: "200px" }}
iconStyle={{ color: "blue" }}
headerStyle={{ backgroundColor: "#f4f4f4", padding: "10px" }}
/>
To prevent edits, set readOnly to true:
<TextEditor readOnly={true} />
TextEditorProps
Prop | Type | Default | Description |
---|---|---|---|
iconStyle | React.CSSProperties | {} | Customize the style of toolbar icons. |
contentStyle | React.CSSProperties | { border: '1px solid #ccc', padding: '10px' } | Customize the editor's content area style. |
headerStyle | React.CSSProperties | {} | Customize the style of the toolbar container. |
placeholder | string | "Type here..." | Placeholder text shown in the editor when it's empty. |
onChange | (e: { target: { value: string } }) => void | undefined | Callback triggered when the editor's content changes. |
readOnly | boolean | false | Sets the editor to read-only mode. |
toolbarActions | Array<string> | See below | Specify the available toolbar actions. |
You can customize the toolbarActions
prop to include any of the following options:
"bold"
: Toggle bold formatting."italic"
: Toggle italic formatting."underline"
: Toggle underline formatting."unorderedList"
: Insert a bulleted list."orderedList"
: Insert a numbered list."alignLeft"
: Align text to the left."alignCenter"
: Align text to the center."alignRight"
: Align text to the right."capitalize"
: Toggle text capitalization."clear"
: Clear all formatting.FAQs
A simple text editor component with formatting options using React.
The npm package react-texteditor-toolkit receives a total of 1 weekly downloads. As such, react-texteditor-toolkit popularity was classified as not popular.
We found that react-texteditor-toolkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.