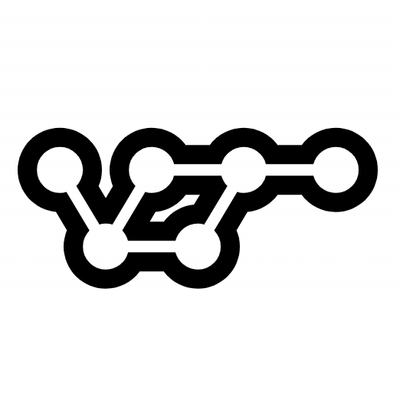
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
simple-deployer
Advanced tools
Commans excecuter that can be use to do a simple deploy or other simple server tasks.
Simple-deployer enables you to execute a series of commands both locally and remotely via SSH. Deploy your application, backup a database or simply get the status of your server with a simple array of commands.
$ npm install simple-deployer --save-dev
// Require simple deployer
const Deployer = require('simple-deployer')
// Server configuration
const config = {
host: 'github.com',
port: 22,
username: 'david'
}
// New deployer
const deployer = new Deployer(config)
// Array of commands
const commands = []
// Push a local command
commands.push({
command: 'cd ~/pictures && ls -l',
local: true
})
// Push a remote command
commands.push({
command: 'cd ~/server_pictures && ls -l',
})
// Deploy
deployer.deploy(commands)
Option | Default | Description |
---|---|---|
host | undefined | domain or ip to be used in the ssh connection |
username | undefined | username to be used in the ssh connection |
port | 22 | port number to be used in the ssh connection |
privateKeyPath | ~/.ssh/id_rsa | path to the private key file to be used in the ssh connection |
password | undefined | server user password to be used in the ssh connection |
showDeployMessages | false | Show extra deployment information |
Option | Type | Description |
---|---|---|
header | String | If present it just print the header string and group the commands after it |
command | String | Command to be executed |
dynamic | function(lastResult: String, lastCode: Number): {command: String, options: Array} | Function to dynamically generate the next command based on results during the deploy |
options | Array | Array of command options like ['-v'] |
local | Boolean | The command must be executed locally |
showResults | Boolean | Print the stdout generated by the command after executing it |
message | String | Custom message to print while executing the command |
continueOnErrorCode | Boolean | Don't stop the deploy if this command returns an error code |
const deployer = new Deployer(config)
const commands = []
commands.push({
command: 'service nginx status',
message: 'nginx status:',
showResults: true
})
deployer.deploy(commands)
Will show:
01 service nginx status
01 nginx status:
● nginx.service - A high performance web server and a reverse proxy server
Loaded: loaded (/lib/systemd/system/nginx.service; enabled; vendor preset: enabled)
Active: active (running) since Tue 2017-08-08 06:44:16 UTC; 1 weeks 0 days ago
Process: 1551 ExecStart=/usr/sbin/nginx -g daemon on; master_process on; (code=exited, status=0/SUCCESS)
Process: 1351 ExecStartPre=/usr/sbin/nginx -t -q -g daemon on; master_process on; (code=exited, status=0/SUCCESS)
Main PID: 1605 (nginx)
Tasks: 28
Memory: 145.1M
CPU: 29min 8.372s
CGroup: /system.slice/nginx.service
├─ 1578 Passenger watchdog
├─ 1586 Passenger core
├─ 1592 Passenger ust-router
├─ 1605 nginx: master process /usr/sbin/nginx -g daemon on; master_process on
├─ 1611 nginx: worker process
└─11046 Passenger RubyApp: /home/deploy/rdi-brinson/current/public (production
✔ 01 david@github.com 0.299s
const deployer = new Deployer(config)
const commands = []
commands.push({
header: 'StaticPage:Create'
})
commands.push({
command: 'echo "<htlm><body>Test Page</body></html>" > index.html',
local: true
})
commands.push({
header: 'StaticPage:Transfer'
})
commands.push({
command: 'mkdir -p sites/github',
})
commands.push({
command: 'scp index.html david@github.com:~/sites/github/',
local: true
})
commands.push({
header: 'StaticPage:CleanUp'
})
commands.push({
command: 'rm index.html',
local: true
})
deployer.deploy(commands)
Will show:
00:00 StaticPage:Create
01 echo "<htlm><body>Test Page</body></html>" > index.html
✔ 01 local 0.215s
00:00 StaticPage:Transfer
01 mkdir -p sites/github
✔ 01 david@github.com 0.297s
02 scp index.html david@github:~/sites/github/
✔ 02 local 1.789s
00:02 StaticPage:CleanUp
01 rm index.html
✔ 01 local 0.165s
const deployer = new Deployer(config)
const commands = []
releases_path = '/home/deploy/apps/github/releases'
commands.push({
header: 'Deploy:Remove oldest'
})
commands.push({
command: ['ls', '-dtr', releases_path + '/*'].join(' '),
showResults: true
})
commands.push({
dynamic: function(lastResult, code) {
var oldestDirectory = lastResult.split('\n')[0]
return { command: ['rm -rf', oldestDirectory].join(' ') }
}
})
deployer.deploy(commands)
Will show:
00:00 Deploy:Remove oldest
01 ls -dtr /home/deploy/apps/github/releases/*
/home/deploy/apps/github/releases/20170808-01
/home/deploy/apps/github/releases/20170808-02
/home/deploy/apps/github/releases/20170809-01
/home/deploy/apps/github/releases/20170809-02
/home/deploy/apps/github/releases/20170809-03
✔ 01 david@github,com 0.341s
02 rm -rf /home/deploy/apps/github/releases/20170808-01
✔ 02 david@github.com 0.299s
MIT
FAQs
Commans excecuter that can be use to do a simple deploy or other simple server tasks.
The npm package simple-deployer receives a total of 0 weekly downloads. As such, simple-deployer popularity was classified as not popular.
We found that simple-deployer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.