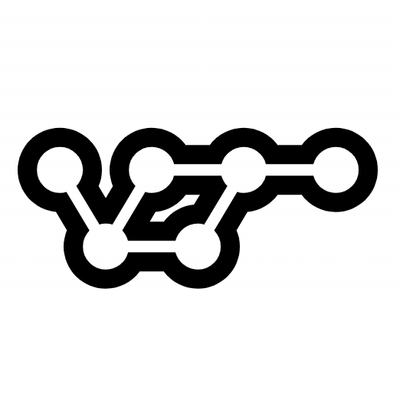
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
snackstack
Advanced tools
Easy-to-use extension of Material-UI, which allows the stacking of Snackbar notification messages.
Easy-to-use extension of Material-UI, which allows the stacking of Snackbar notification messages.
Method | Command |
---|---|
npm | npm install snackstack |
yarn | yarn add snackstack |
Wrap all components, which should be able to enqueue or close Snackbar Notifications, in a SnackProvider
:
import { SnackProvider } from 'snackstack';
ReactDOM.render(
<SnackProvider>
<App />
</SnackProvider>,
document.getElementById('root'),
);
If you're using MuiThemeProvider
make sure that you place the SnackProvider
inside of it.
The withSnacks
HOC injects the enqueueSnack
and closeSnack
function into your component's props
:
import { withSnacks } from 'snackstack';
class ExampleComponent extends React.Component {
handleEnqueueClick = () => {
const { enqueueSnack } = this.props;
enqueueSnack({ message: 'Hello World', key: 'key123' });
};
handleCloseClick = () => {
const { closeSnack } = this.props;
closeSnack('key123');
};
render() {
return (
<div>
<button onClick={this.handleEnqueueClick}>Enqueue</button>
<button onClick={this.handleCloseClick}>Close</button>
</div>
);
}
}
export default withSnacks(ExampleComponent);
The useSnacks
Hook returns an array containing the enqueueSnack
and closeSnack
function:
import { useSnacks } from 'snackstack';
const ExampleComponent = () => {
const [enqueueSnack, closeSnack] = useSnacks();
const handleEnqueueClick = () => {
enqueueSnack({ message: 'Hello World', key: 'key123' });
};
const handleCloseClick = () => {
closeSnack('key123');
};
return (
<div>
<button onClick={handleEnqueueClick}>Enqueue</button>
<button onClick={handleCloseClick}>Close</button>
</div>
);
};
export default ExampleComponent;
If you're unfamiliar with Hooks I suggest this article as an introduction.
Not yet available
FAQs
Easy-to-use extension of Material-UI, which allows the stacking of Snackbar notification messages.
The npm package snackstack receives a total of 0 weekly downloads. As such, snackstack popularity was classified as not popular.
We found that snackstack demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.