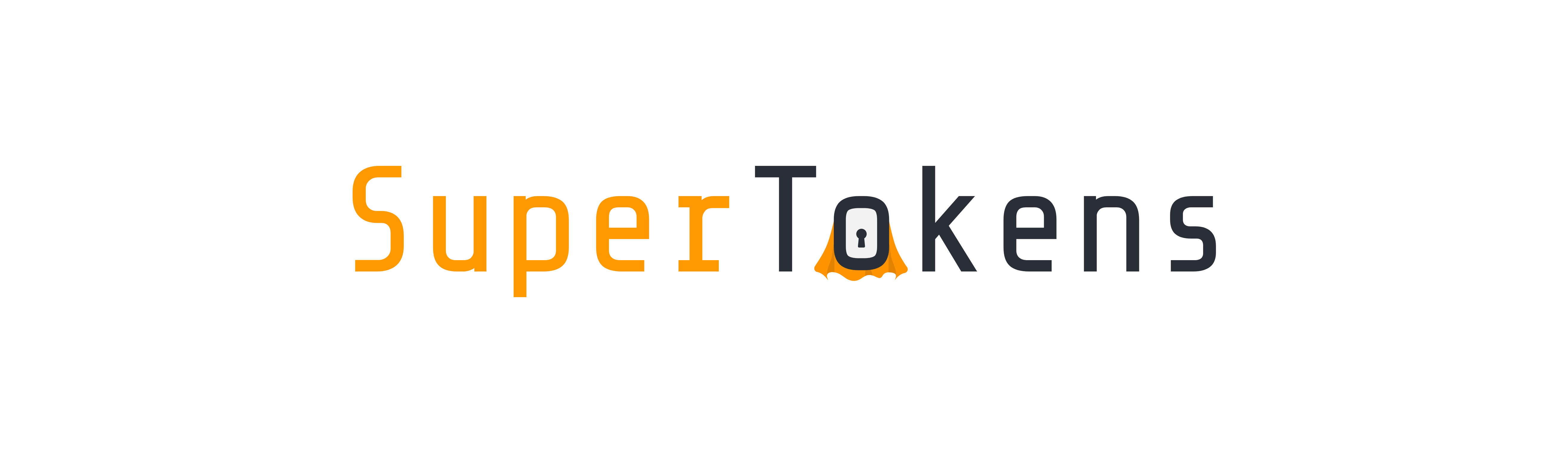
SuperTokens Javascript Frontend SDK
SuperTokens adds secure login and session management to your apps. This is the Javascript Frontend SDK for SuperTokens. More SDKs are available for frontend and backend e.g. Node.js, Go, Python, React.js, React Native, Vanilla JS, etc.
SuperTokens architecture is optimized to add secure authentication for your users without compromising on user and developer experience
Learn more at supertokens.com
How to install
Using npm
npm i -s supertokens-website
OR simply add the following script tag to your HTML pages
<script src="https://cdn.jsdelivr.net/gh/supertokens/supertokens-website/bundle/bundle.js"></script>
How to use
- Initialize SuperTokens in your frontend
supertokens.init({
apiDomain: "<URL to your auth backend>"
});
- Make sure your backend has the needed auth functionalities
You can use one of the SuperTokens backend SDKs for this.
Backend SDKs are available for
Now that's the basic setup. But you might want to do some of the following things
Checking if a session exists
await supertokens.doesSessionExist();
Reading the userId
let userId = await supertokens.getUserId();
Reading the access token payload
let payload = await supertokens.getAccessTokenPayloadSecurely();
Sign out
The signOut method simply revokes the session on the frontend and backend.
await supertokens.signOut();
Sending requests with fetch
The init
function call automatically adds interceptors to fetch. So there is nothing else that needs to be done.
supertokens.init({
apiDomain: "https://api.example.com"
});
async function doAPICalls() {
try {
let fetchConfig = { ... };
let response = await fetch("/someAPI", fetchConfig);
if (response.status !== 200) {
throw response;
}
let data = await response.json();
let someField = data.someField;
} catch (err) {
if (err.status === 401) {
} else {
}
}
}
Sending requests with axios
supertokens.addAxiosInterceptors(axios);
supertokens.init({
apiDomain: "https://api.example.com"
});
async function doAPICalls() {
try {
let postData = { ... };
let response = await axios({url: "someAPI", method: "post", data: postData });
let data = await response.data;
let someField = data.someField;
} catch (err) {
if (err.response !== undefined && err.response.status === 401) {
} else {
}
}
}
Documentation
To see documentation, please click here.
Contributing
Please refer to the CONTRIBUTING.md file in this repo.
Contact us
For any queries, or support requests, please email us at team@supertokens.com, or join our Discord server.
Authors
Created with :heart: by the folks at SuperTokens.com