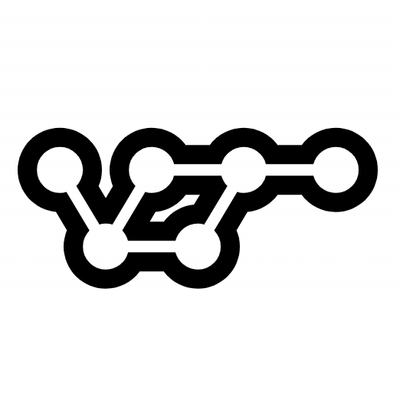
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
swift-playground-builder
Advanced tools
Create Xcode Playgrounds for the Swift programming language with rich documentation generated from Markdown
Create Xcode Playgrounds for the Swift programming language with rich documentation generated from Markdown
Swift code is extracted from the Markdown with the same syntax used to specify languages for code blocks in GitHub-flavored Markdown. All other text is parsed normally as Markdown using marked.
# A Swift Tour
Tradition suggests that the first program in a new language should print the words "Hello, world"
on the screen. In Swift, this can be done in a single line:
```swift
println("Hello, world!")
```
This tool requires Node.js. Download the installer from the Node.js website and follow the instructions.
In your Terminal, run the following command to install the Playground Builder:
$ npm install -g swift-playground-builder
That's it! You should now be able to use the playground
command.
Usage: playground <paths>... [options]
paths Markdown files(s), or directory containing Markdown files,
from which to build the Playground(s)
Options:
-d, --destination Directory in which to output the Playground(s)
-p, --platform Specifies which platform's frameworks can be imported
in the Playground(s); only one platform can be choosen
[options: ios, osx] [default: osx]
-n, --noreset Don't allow edited code to be reset from the
"Editor → Reset Playground" menu in Xcode
-v, --version Print swift-playground-builder version and exit
You can also import the Playground Builder as a Node.js module.
var buildPlayground = require('swift-playground-builder');
paths
(String
or Array
, required)
Path to Markdown file or directory containg Markdown files. An array of file and/or directory paths is also acceptable.
outputDirectory
(String
, optional)
Path to directory in which to output the built Playground(s). If not specified, the value of process.cwd()
is used by default.
options
(Object
, optional)
See the "Options" section below for available options.
callback
(Function
, optional)
Function to be called once all Playground files have been output. First argument is err
which contains an Error
, if any.
allowsReset
(default: true
)
A Playground's code can be modified and saved. The Playground can be reset to its original code from the "Editor → Reset Playground" menu. This menu can be disabled for a Playground by setting this option to false
.`
platform
(default: osx
)
Set the platform to osx
or ios
to be able to import each platform's respective frameworks.
var buildPlayground = require('swift-playground-builder');
// outputs `Introduction.playground` to CWD
buildPlayground('Introduction.md');
// outputs `Variables.playground` to `/User/json/Playgrounds`
buildPlayground('Variables.md', '/User/jason/Playgrounds');
// outputs `Constants.playground` and `Closures.playground` to CWD
buildPlayground(['Constants.md', 'Closures.md'], {
allowsReset: false,
platform: 'ios'
});
// outputs playgrounds for Markdown files in `./playgrounds` directory,
// then invokes callback function
buildPlayground(['./playgrounds'], function(err) {
if (err) { throw err; }
console.log('Done building playgrounds!');
});
FAQs
> Create Xcode Playgrounds for the Swift programming language with rich documentation generated from Markdown
The npm package swift-playground-builder receives a total of 0 weekly downloads. As such, swift-playground-builder popularity was classified as not popular.
We found that swift-playground-builder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.