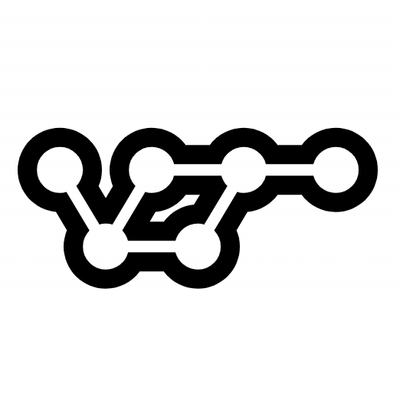
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
telegram-bot-mark-loku
Advanced tools
1 - Create a new JavaScript file in your project directory. You can name it something like bot.js.
2 - Open the bot.js file in a code editor of your choice.
3 - Begin by requiring the npm i telegram-bot-loku package at the top of your file:
#CODE:
const TelegramBot = require('telegram-bot-loku');
4 - Initialize the bot by passing your API token and enabling the polling option. This allows the bot to listen for and receive new messages:
#CODE:
const token = 'YOUR_TELEGRAM_BOT_TOKEN'; // Replace with your own bot token
const channel = 'YOUR_TELEGRAM_BOT_CHANNEL'; // Replace with your own bot channel
const message = 'Hi Everyone'; // Replace with your message
async function sendMessage() {
try {
const telegramResponse = await telegramInstance.sendMessage("token", "channel", "Hi");
console.log(telegramResponse);
return telegramResponse;
} catch (error) {
console.error('Error:', error);
throw error; // Re-throw the error if necessary
}
}
5 - Add an event listener to handle incoming messages. This listener will be triggered whenever a user sends a message to your bot:
#CODE:
sendMessage()
.then(response => {
console.log('Success:', response);
})
.catch(error => {
console.error('Error:', error);
});
By following the previous steps, your bot.js file will look like this:
#CODE:
const telegramInstance = require('telegram-bot-loku')
async function sendMessage() {
try {
const telegramResponse = await telegramInstance.sendMessage("token", "channel", "Hi");
console.log(telegramResponse);
return telegramResponse;
} catch (error) {
console.error('Error:', error);
throw error; // Re-throw the error if necessary
}
}
sendMessage().catch(error => {
console.error('Error:', error);
});
1 - Open a terminal or command prompt and navigate to your project directory.
2 - Run the command node bot.js to start the bot. You should see a message indicating that the bot is running and waiting for incoming messages.
3 - Switch to the Telegram app on your device and find your bot by its username or display name.
4 - Send a message to your bot and observe its response. If you implemented the /start command, you should receive a welcome message.
FAQs
This npm package is a lightweight and efficient solution designed to streamline the process of sending messages via Telegram Bot API. Whether you're a developer building a sophisticated bot or an enthusiast experimenting with Telegram's capabilities, this
We found that telegram-bot-mark-loku demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.