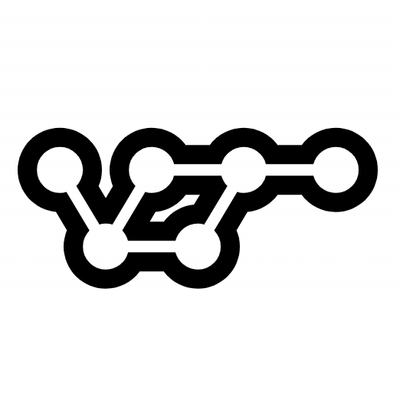
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
upload-js
Advanced tools
JavaScript File Upload Library
(With Integrated Cloud Storage)
Files are hosted on Upload.io: the file upload service for developers.
Install via NPM:
npm install upload-js
Or via NPM:
yarn add upload-js
Or via a <script>
tag:
<script src="https://js.upload.io/upload-js/v1"></script>
<input>
element β Try on CodePenTo create a working file upload button, copy this example:
<html>
<head>
<script src="https://js.upload.io/upload-js/v1"></script>
<script>
const upload = new Upload({
// Get production API keys from Upload.io
apiKey: "free"
});
const uploadFile = upload.createFileInputHandler({
onProgress: ({ bytesSent, bytesTotal }) => {
console.log(`${bytesSent / bytesTotal}% complete`)
},
onUploaded: ({ fileUrl, fileId }) => {
alert(`File uploaded!\n${fileUrl}`);
},
onError: (error) => {
alert(`Error!\n${error.message}`);
}
});
</script>
</head>
<body>
<input type="file" onchange="uploadFile(event)" />
</body>
</html>
File
object β Try on CodePenIf you have a File
object already, use the upload.uploadFile(...)
method:
const { Upload } = require("upload-js");
const upload = new Upload({ apiKey: "free" });
const onFileInputChange = async event => {
const fileObject = event.target.files[0];
const { fileUrl, fileId } = await upload.uploadFile({
file: fileObject,
onProgress: ({ bytesSent, bytesTotal }) => {
console.log(`${bytesSent / bytesTotal}% complete`)
}
});
alert(`File uploaded to: ${fileUrl}`);
}
Uploader is our file & image upload widget, powered by Upload.js.
Uploader has a larger payload size (29kB) compared to Upload.js (7kB), but if you're writing a file upload UI component, it could save you time: Uploader provides things like progress bars and cancellation out-the-box.
const { Upload } = require("upload-js");
const upload = new Upload({ apiKey: "free" });
const MyUploadButton = () => {
const uploadFile = upload.createFileInputHandler({
onProgress: ({ bytesSent, bytesTotal }) => {
console.log(`${bytesSent / bytesTotal}% complete`)
},
onUploaded: ({ fileUrl, fileId }) => {
alert(`File uploaded!\n${fileUrl}`);
},
onError: (error) => {
alert(`Error!\n${error.message}`);
}
});
return <input type="file" onChange={uploadFile} />;
};
const { Upload } = require("upload-js");
const upload = new Upload({ apiKey: "free" });
angular
.module("exampleApp", [])
.controller("exampleController", $scope => {
$scope.uploadFile = upload.createFileInputHandler({
onProgress: ({ bytesSent, bytesTotal }) => {
console.log(`${bytesSent / bytesTotal}% complete`)
},
onUploaded: ({ fileUrl, fileId }) => {
alert(`File uploaded!\n${fileUrl}`);
},
onError: (error) => {
alert(`Error!\n${error.message}`);
}
});
})
.directive("onChange", () => ({
link: (scope, element, attrs) => {
element.on("change", scope.$eval(attrs.onChange));
}
}));
const { Upload } = require("upload-js");
const upload = new Upload({ apiKey: "free" });
const uploadFile = upload.createFileInputHandler({
onProgress: ({ bytesSent, bytesTotal }) => {
console.log(`${bytesSent / bytesTotal}% complete`)
},
onUploaded: ({ fileUrl, fileId }) => {
alert(`File uploaded!\n${fileUrl}`);
},
onError: (error) => {
alert(`Error!\n${error.message}`);
}
});
const vueApp = new Vue({
el: "#example",
methods: { uploadFile }
});
<html>
<head>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://js.upload.io/upload-js/v1"></script>
<script>
const upload = new Upload({
// Get production API keys from Upload.io
apiKey: "free"
});
$(() => {
$("#file-input").change(
upload.createFileInputHandler({
onBegin: () => {
$("#file-input").hide()
},
onProgress: ({ bytesSent, bytesTotal }) => {
const progress = Math.round(bytesSent / bytesTotal * 100)
$("#title").html(`File uploading... ${progress}%`)
},
onError: (error) => {
$("#title").html(`Error:<br/><br/>${error.message}`)
},
onUploaded: ({ fileUrl, fileId }) => {
$("#title").html(`
File uploaded:
<br/>
<br/>
<a href="${fileUrl}" target="_blank">${fileUrl}</a>`
)
}
})
)
})
</script>
</head>
<body>
<h1 id="title">Please select a file...</h1>
<input type="file" id="file-input" />
</body>
</html>
Please refer to the CodePen example (link above).
Overview of the code:
Upload
once in your app (at the start).createFileInputHandler
once for each file <input>
element.onProgress
to display the upload progress for each input element.onUploaded
fires, record the fileUrl
from the callback's argument to a local variable.onUploaded
has fired for all files, the form is ready to be submitted.Note: file uploads will safely run in parallel, despite using the same Upload
instance.
By default, the browser will attempt to render uploaded files:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ
To force a file to download, add ?download=true
to the file's URL:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ?download=true
Given an uploaded image URL:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ
Resize with:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ/thumbnail
Auto-crop with:
https://upcdn.io/W142hJkHhVSQ5ZQ5bfqvanQ/thumbnail-square
Tip: to create more transformations, please register an account.
To crop images using manually-provided geometry:
<html>
<head>
<script src="https://js.upload.io/upload-js/v1"></script>
<script>
const upload = new Upload({
// Get production API keys from Upload.io
apiKey: "free"
});
// Step 1: Upload the original file.
const onOriginalImageUploaded = ({ fileId, fileUrl: originalImageUrl }) => {
// Step 2: Configure crop geometry.
const crop = {
// Type Def: https://github.com/upload-js/upload-image-plugin/blob/main/src/types/ParamsFromFile.ts
input: fileId,
pipeline: {
steps: [
{
geometry: {
// Prompt your user for these dimensions...
offset: {
x: 20,
y: 40
},
size: {
// ...and these too...
width: 200,
height: 100,
type: "widthxheight!"
}
},
type: "crop"
}
]
}
}
// Step 3: Upload the crop geometry.
const blob = new Blob([JSON.stringify(crop)], {type: "application/json"});
upload
.uploadFile({
file: {
name: `${fileId}_cropped.json`, // Can be anything.
type: blob.type,
size: blob.size,
slice: (start, end) => blob.slice(start, end)
}
})
.then(
({ fileUrl: cropGeometryUrl }) => {
// Step 4: Done! Here's the cropped image's URL:
alert(`Cropped image:\n${cropGeometryUrl}/thumbnail`)
},
e => console.error(e)
);
};
const uploadFile = upload.createFileInputHandler({
onUploaded: onOriginalImageUploaded
});
</script>
</head>
<body>
<input type="file" onchange="uploadFile(event)" />
</body>
</html>
See Upload.js Documentation Β»
Upload.js is the JavaScript client library for Upload.io: the file upload service for developers.
Core features:
"free"
API key.)Available with an account:
"free"
API key provides temporary storage only.)"free"
API key allows 100 uploads per day per IP.)Create an Upload.io account Β»
If you would like to contribute to Upload.js:
FAQs
File Upload Library β Upload.js gives developers AJAX multipart file uploading via XHR π Comes with Cloud Storage π
The npm package upload-js receives a total of 7,542 weekly downloads. As such, upload-js popularity was classified as popular.
We found that upload-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezerβs API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.