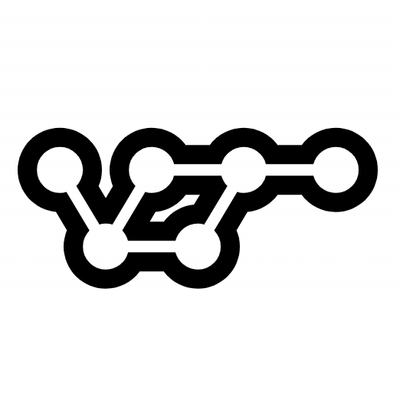
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
vite-plugin-r3f-transform
Advanced tools
A Vite plugin for React Three Fiber that enables scene editing capabilities. The plugin provides transform controls for meshes and automatically saves their transformations to a JSON file.
A Vite plugin for React Three Fiber that enables scene editing capabilities. The plugin provides transform controls for meshes and automatically saves their transformations to a JSON file.
npm install vite-plugin-r3f-transform
// vite.config.js
import r3fTransform from 'vite-plugin-r3f-transform'
export default {
plugins: [r3fTransform()]
}
DevTransformWrapper
in your R3F scene:import { DevTransformWrapper } from 'virtual:r3f-transform'
function Scene() {
return (
<DevTransformWrapper>
<mesh name="uniqueName">
<boxGeometry />
<meshStandardMaterial />
</mesh>
</DevTransformWrapper>
)
}
name
prop// Good
<mesh name="cube1">...</mesh>
<mesh name="cube2">...</mesh>
// Bad - Missing name
<mesh>...</mesh>
// Bad - Duplicate name
<mesh name="cube1">...</mesh>
<mesh name="cube1">...</mesh>
Transformations are automatically saved to public/transform-data.json
in the following format:
{
"meshName1": {
"position": [x, y, z],
"rotation": [x, y, z],
"scale": [x, y, z]
},
"meshName2": {
"position": [x, y, z],
"rotation": [x, y, z],
"scale": [x, y, z]
}
}
Escape
to deselect the current meshDevTransformWrapper
componenttransform-data.json
To modify or extend the plugin:
The plugin consists of two main parts:
Key files:
vite-plugin-r3f-transform.js
: Main plugin filetransform-data.json
: Generated file storing transformationsPotential areas for enhancement:
Feel free to submit issues and enhancement requests!
MIT License
FAQs
A Vite plugin for React Three Fiber that enables scene editing capabilities. The plugin provides transform controls for meshes and automatically saves their transformations to a JSON file.
The npm package vite-plugin-r3f-transform receives a total of 1 weekly downloads. As such, vite-plugin-r3f-transform popularity was classified as not popular.
We found that vite-plugin-r3f-transform demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.