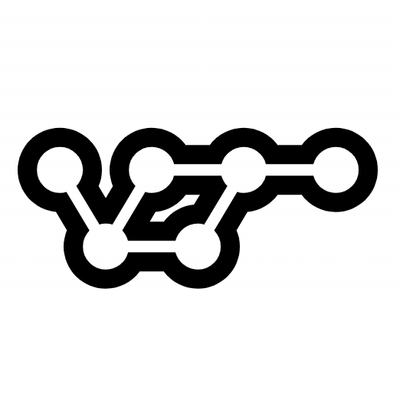
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
vue-native-notification
Advanced tools
Vue.js plugin for native notifications
npm install --save vue-native-notification
import Vue from 'vue'
import VueNativeNotification from 'vue-native-notification'
Vue.use(VueNativeNotification, {
// Automatic permission request before
// showing notification (default: true)
requestOnNotify: true
})
<template>
<button type="button" @click="notify">Show notification</button>
</template>
<script>
export default {
methods: {
notify () {
// https://developer.mozilla.org/en-US/docs/Web/API/Notification/Notification#Parameters
this.$notification.show('Hello World', {
body: 'This is an example!'
}, {})
}
}
}
</script>
<style>
</style>
You can manually request users permission with:
// Global
Vue.notification.requestPermission()
.then(console.log) // Prints "granted", "denied" or "default"
// Component
this.$notification.requestPermission()
.then(console.log)
https://developer.mozilla.org/en-US/docs/Web/API/Notification
We now supports all notifications events
https://developer.mozilla.org/en-US/docs/Web/API/Notification/onerror
Is an empty function. Nothing will be executed
https://developer.mozilla.org/en-US/docs/Web/API/Notification/onclick
When notification is clicked, we set the focus on the context browser and close the notification
https://developer.mozilla.org/en-US/docs/Web/API/Notification/onclose
Is an empty function. Nothing will be executed
https://developer.mozilla.org/en-US/docs/Web/API/Notification/onshow
Is an empty function. Nothing will be executed
const notification = {
title: 'Your title',
options: {
body: 'This is an example!'
},
events: {
onerror: function () {
console.log('Custom error event was called');
},
onclick: function () {
console.log('Custom click event was called');
},
onclose: function () {
console.log('Custom close event was called');
},
onshow: function () {
console.log('Custom show event was called');
}
}
}
this.$notification.show(notification.title, notification.options, notification.events)
FAQs
Vue.js plugin for native notifications
The npm package vue-native-notification receives a total of 901 weekly downloads. As such, vue-native-notification popularity was classified as not popular.
We found that vue-native-notification demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.