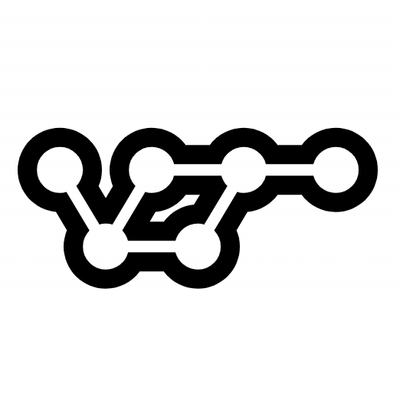
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Welcome to Docusearch, the ultimate tool for document searching and processing using the power of vector embeddings. Follow the steps below to get started quickly!
First, you need to install the docusearch
package using pip
. Installation may take a minute or two. To avoid conflicts, it is recommend you use a virtual environment:
pip install docusearch
After installing the package, import the process_query
function from the docusearch
module:
from docusearch import process_query
Define the parameters for your query. You need to set your OpenAI API key, the path to the folder containing the documents, and the query for those documents
query = "What are some cool features of the Audi r8"
api_key = "your-openai-key"
folder_path = "path-to-your-folder"
Now, call the process_query
function with the parameters you set and print the result:
result = process_query(query, api_key, folder_path)
print(result)
Here is the complete example code:
from docusearch import process_query
query = "What are some cool features of the Audi r8"
api_key = "your-openai-key"
folder_path = "path-to-your-folder"
result = process_query(query, api_key, folder_path)
print(result)
And that's it! You have successfully used the docusearch package to process your query. Enjoy searching your documents with ease!
If you would like specific information about the result, you can use the extract_info
function. It will provide you the document source, answer, citations, and warnings for unsupported files.
from docusearch import extract_info
document_source, answer, citations, warning = extract_info(result)
print(f"Document Source: {document_source}")
print(f"Answer: {answer}")
print(f"Citations: {citations}")
if warning:
print(f"Warning: {warning}")
Here is the complete example code to query and extract specific information:
from docusearch import process_query, extract_info
query = "What are some cool features of the Audi r8"
api_key = "your-openai-key"
folder_path = "path-to-your-folder"
# Process the query
result = process_query(query, api_key, folder_path)
# Extract information from the result
document_source, answer, citations, warning = extract_info(result)
# Print the extracted information
print(f"Document Source: {document_source}")
print(f"Answer: {answer}")
print(f"Citations: {citations}")
if warning:
print(f"Warning: {warning}")
By following these steps, you can easily extract and use specific pieces of information from the result provided by the docusearch
package. Have fun!
FAQs
Query documents quickly and efficiently
We found that docusearch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.