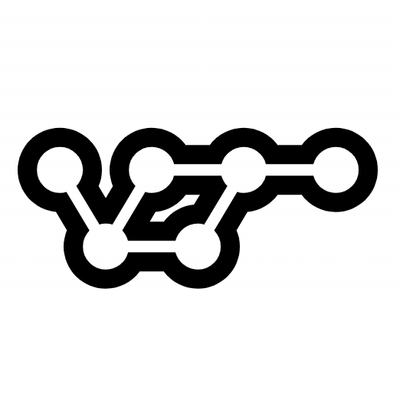
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
This module provides a framework for handling and processing asynchronous tasks through message queues.
This module provides a framework for handling and processing asynchronous tasks through message queues.
To define a task, instantiate the MqTasks object and use the task decorator:
import mqtasks
mq_tasks = MqTasks(amqp_connection="amqp://localhost", queue_name="my_queue")
@mq_tasks.task(name="my_task")
def my_task_function(ctx: MqTaskContext):
# Process task
pass
mq_tasks.run()
Instantiate MqTasksClient and use it to send a task:
import asyncio
import mqtasks
client = MqTasksClient(
loop=asyncio.get_event_loop(),
amqp_connection="amqp://localhost"
)
# define channel
channel = await client.queue(queue_name="my_queue")
#
result = await channel.request_task_async(task_name="my_task", body={"message": "hello world"})
./example
cd ./example
docker pull rabbitmq:management
docker run -d --name rabbitmq -p 5672:5672 -p 15672:15672 rabbitmq:management
http://localhost:15672/
python example_server.py
python example_client.py
make a release/x.x.x branch, up the version and commit
change the minor version 0.X.0
./scripts/release.sh minor
change the patch version 0.0.X
./scripts/release.sh patch
merge the release/x.x.x branch into the master and the develop branch
./scripts/merge.sh
MIT License
FAQs
This module provides a framework for handling and processing asynchronous tasks through message queues.
We found that mqtasks demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.