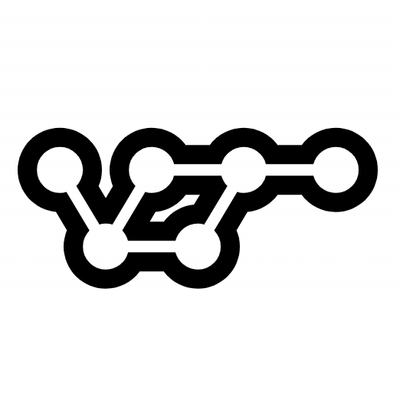
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
A Python library for loading stock data from the Seeking Alpha API.
You can install the Stock Data Loader using pip:
pip install stock-data-loader
The StockDataLoader
class provides an easy way to fetch and process stock data from the Seeking Alpha API. Here's a quick guide on how to use it:
Import the class:
from stock_data_loader import StockDataLoader
Create an instance of the loader:
loader = StockDataLoader()
Prepare a list of stock symbols you want to fetch data for:
symbols = ['AAPL', 'GOOGL', 'MSFT', 'AMZN']
Use the load_symbol_data
method to fetch and process the data:
result_df = loader.load_symbol_data(symbols)
The result is a pandas DataFrame. You can now work with this data:
print(result_df)
Example:
from stock_data_loader import StockDataLoader
loader = StockDataLoader()
symbols = ['AAPL', 'GOOGL', 'MSFT', 'AMZN']
result_df = loader.load_symbol_data(symbols)
# Print the first few rows of the result
print(result_df.head())
# Save the result to a CSV file
result_df.to_csv('stock_data.csv', index=False)
This will fetch data for the specified symbols, process it, and return a DataFrame with various attributes like symbol, name, follower count, exchange, and content counters for analysis, news, transcripts, etc.
The load_symbol_data
method returns a pandas DataFrame with the following columns:
id
: Unique identifier for the stocktype
: Type of the data (usually "ticker")symbol
: Stock symbolname
: Full name of the companyfollowersCount
: Number of followers on Seeking Alphaexchange
: Stock exchange where the stock is listedanalysis
: Number of analysis articlesrelated_analysis
: Number of related analysis articlestranscripts
: Number of earnings call transcriptsearning_slides
: Number of earning slides availablenews
: Number of news articlespartnerNews
: Number of partner news articlespressReleases
: Number of press releasesbulls_say
: Number of bullish opinionsbears_say
: Number of bearish opinionsinvesting_groups
: Number of investing groups discussing the stockannual_dividends
: Number of annual dividend reportsannual_earnings_estimates
: Number of annual earnings estimatesdividend_news
: Number of dividend-related news itemssec_filings
: Number of SEC filingssec_filings_fin_and_news
: Number of financial and news-related SEC filingssec_filings_tenders
: Number of tender offer SEC filingssec_filings_other
: Number of other SEC filingssec_filings_ownership
: Number of ownership-related SEC filingssector_rating_change_notices
: Number of sector rating change noticessector_quant_warnings
: Number of quantitative warnings for the sectorsector_dividend_safety_warnings
: Number of dividend safety warnings for the sectorquarterly_revenue
: Number of quarterly revenue reportsannual_revenue
: Number of annual revenue reportsmarket_open
: Market open statusmarket_open_time
: Market open timeanalysis_count
: Another count of analysis articles (may differ from analysis
)news_count
: Another count of news articles (may differ from news
)transcripts_count
: Another count of transcripts (may differ from transcripts
)Note: Some columns may be empty or have different values than expected due to variations in the API response.
Here's a sample of what the output might look like:
print(result_df[['symbol', 'followersCount', 'analysis', 'news', 'sec_filings', 'annual_dividends']].head())
symbol followersCount analysis news sec_filings annual_dividends
0 AAPL 2713202 10037 10753 121 0
1 TSLA 1151910 5929 5737 121 0
2 GOOGL 459787 1974 4592 97 0
Contributions are welcome! Please feel free to submit a Pull Request.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
A Python library for loading stock data from the Seeking Alpha API
We found that stock-data-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.