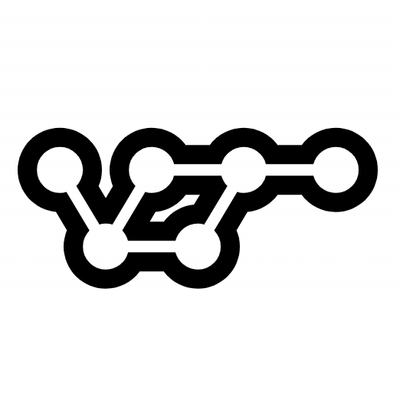
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
availability_scheduler
Advanced tools
AvailabilityScheduler
is a Ruby on Rails gem for managing user availability and scheduling appointments. It ensures appointments are booked within the user's available time slots and prevents overlapping bookings. The gem supports both recurring weekly schedules and one-off special schedules.
Add this line to your application's Gemfile
:
gem 'availability_scheduler'
And then execute:
bundle install
Next, run the generator to create the necessary migration files:
rails generate availability_scheduler:install
Finally, migrate your database:
rails db:migrate
Define recurring weekly schedules for users.
# Define a weekly schedule for a user
AvailabilityScheduler::WeeklySchedule.create!(
user_id: 1,
day_of_week: 1, # Monday
start_time: '09:00',
end_time: '17:00'
)
Define one-off special schedules for specific dates.
# Define a special schedule for a specific date
AvailabilityScheduler::SpecialSchedule.create!(
user_id: 1,
date: '2024-09-10',
start_time: '10:00',
end_time: '14:00'
)
Book an appointment with validation to ensure it’s within the user’s availability.
AvailabilityScheduler::Appointment.create!(
booker_id: 2, # User making the booking
booked_user_id: 1, # User being booked
date: '2024-09-10',
start_time: '11:00',
end_time: '12:00'
)
You can fetch the availability of a user for a given month using the AvailabilityScheduler::Availability::FetchForUser
service. This service returns a hash where each day of the month is mapped to an array of availability time periods.
availability_service = AvailabilityScheduler::Availability::FetchForUser.new(user_id: 1, date: '05-2024')
availability = availability_service.call
availability.each do |date, periods|
if periods.any?
periods.each do |period|
puts "#{date}: Available from #{period[:start_time]} to #{period[:end_time]}"
end
else
puts "#{date}: Not available"
end
end
Example output:
{
"2024-05-01" => [{ start_time: "09:00", end_time: "10:00" }, { start_time: "11:00", end_time: "16:00" }],
"2024-05-02" => [],
"2024-05-03" => [{ start_time: "10:00", end_time: "14:00" }],
# ...
}
The gem will automatically validate whether the appointment is within the availability and if it overlaps with any other appointments.
appointment = AvailabilityScheduler::Appointment.new(
booker_id: 2,
booked_user_id: 1,
date: '2024-09-10',
start_time: '11:00',
end_time: '12:00'
)
if appointment.save
puts 'Appointment successfully booked!'
else
puts appointment.errors.full_messages
end
Bug reports and pull requests are welcome on GitHub at https://github.com/yourusername/availability_scheduler.
This project is available as open source under the terms of the MIT License.
FAQs
Unknown package
We found that availability_scheduler demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.