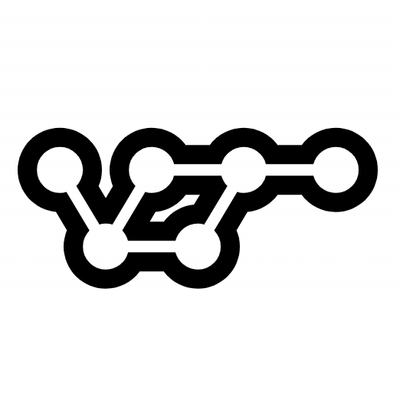
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
This client library is a Ruby gem which can be compiled and used in your Ruby and Ruby on Rails project. This library requires a few gems from the RubyGems repository.
gem build reward_sciences.gemspec
to build the gem.gem install reward_sciences-1.0.gem

The following section explains how to use the RewardSciences Ruby Gem in a new Rails project using RubyMine™. The basic workflow presented here is also applicable if you prefer using a different editor or IDE.
Close any existing projects in RubyMine™ by selecting File -> Close Project
. Next, click on Create New Project
to create a new project from scratch.

Next, provide TestApp
as the project name, choose Rails Application
as the project type, and click OK
.

In the next dialog make sure that correct Ruby SDK is being used (minimum 2.0.0) and click OK
.

This will create a new Rails Application project with an existing set of files and folder.
In order to use the RewardSciences gem in the new project we must add a gem reference. Locate the Gemfile
in the Project Explorer window under the TestApp
project node. The file contains references to all gems being used in the project. Here, add the reference to the library gem by adding the following line: gem 'reward_sciences', '~> 1.0'

Once the TestApp
project is created, a folder named controllers
will be visible in the Project Explorer under the following path: TestApp > app > controllers
. Right click on this folder and select New -> Run Rails Generator...
.

Selecting the said option will popup a small window where the generator names are displayed. Here, select the controller
template.

Next, a popup window will ask you for a Controller name and included Actions. For controller name provide Hello
and include an action named Index
and click OK
.

A new controller class anmed HelloController
will be created in a file named hello_controller.rb
containing a method named Index
. In this method, add code for initialization and a sample for its usage.

You can test the generated SDK and the server with automatically generated test cases as follows:
bundle exec rake
In order to setup authentication and initialization of the API client, you need the following information.
Parameter | Description |
---|---|
o_auth_access_token | The OAuth 2.0 access token to be set before API calls |
API client can be initialized as following.
# Configuration parameters and credentials
o_auth_access_token = "o_auth_access_token"; # The OAuth 2.0 access token to be set before API calls
client = RewardSciences::RewardSciencesClient.new(o_auth_access_token)
The added initlization code can be debugged by putting a breakpoint in the Index
method and running the project in debug mode by selecting Run -> Debug 'Development: TestApp'
.

The singleton instance of the Rewards
class can be accessed from the API Client.
rewards = client.rewards
Bid on a reward auction.
def bid(user_id,
reward_id,
amount); end
Parameter | Tags | Description |
---|---|---|
user_id | Required | The ID of the user who is bidding on the reward auction. |
reward_id | Required | The ID of the reward auction to be bid on. |
amount | Required | Can be either 'max' (when max bidding) or the number of points the user wants to bid. |
user_id = 225
reward_id = 225
amount = 'amount'
result = rewards.bid(user_id, reward_id, amount)
List all the available rewards.
def list(category_id = nil,
limit = 25,
offset = 0); end
Parameter | Tags | Description |
---|---|---|
category_id | Optional | The id of the category to filter rewards by |
limit | Optional DefaultValue | The number of rewards you want to be retrieved. |
offset | Optional DefaultValue | The number of rewards you want to skip before starting the retrieval. |
category_id = 225
limit = 25
offset = 0
result = rewards.list(category_id, limit, offset)
Redeem a reward.
def redeem(user_id,
reward_id); end
Parameter | Tags | Description |
---|---|---|
user_id | Required | The ID of the user who is redeeming the reward. |
reward_id | Required | The ID of the reward to be redeemed. |
user_id = 225
reward_id = 225
result = rewards.redeem(user_id, reward_id)
Show a reward's details.
def show(reward_id); end
Parameter | Tags | Description |
---|---|---|
reward_id | Required | The ID of the reward to be retrieved. |
reward_id = 225
result = rewards.show(reward_id)
The singleton instance of the RewardCategories
class can be accessed from the API Client.
rewardCategories = client.reward_categories
List all the available reward categories.
def list(limit = 25,
offset = 0); end
Parameter | Tags | Description |
---|---|---|
limit | Optional DefaultValue | The number of reward categories you want to be retrieved. |
offset | Optional DefaultValue | The number of reward categories you want to skip before starting the retrieval. |
limit = 25
offset = 0
result = rewardCategories.list(limit, offset)
The singleton instance of the Users
class can be accessed from the API Client.
users = client.users
This endpoint lets retrieve a user's details.
def show(user_id); end
Parameter | Tags | Description |
---|---|---|
user_id | Required | The ID of the user to be retrieved. |
user_id = 225
result = users.show(user_id)
This endpoint lets you tie a user with his/her activities. You’ll want to identify a user with any relevant information as soon as they log-in or sign-up.
def identify(email,
first_name = nil,
last_name = nil); end
Parameter | Tags | Description |
---|---|---|
Required | The user's email address | |
first_name | Optional | The user's first name |
last_name | Optional | The user's last name |
email = 'email'
first_name = 'first_name'
last_name = 'last_name'
result = users.identify(email, first_name, last_name)
The singleton instance of the Activities
class can be accessed from the API Client.
activities = client.activities
This endpoint lets you track the activities your users perform.
def track(user_id,
activity_type,
price = nil,
record_id = nil); end
Parameter | Tags | Description |
---|---|---|
user_id | Required | The ID of the user who is performing the activity. |
activity_type | Required | The type of activity the user is performing. Example: 'purchased-a-product' |
price | Optional | The price related to the activity, if any. Expressed in USD |
record_id | Optional | The ID for the record associated with the activity in your database. |
user_id = 225
activity_type = 'activity_type'
price = 225
record_id = 'record_id'
result = activities.track(user_id, activity_type, price, record_id)
FAQs
Unknown package
We found that reward_sciences demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.