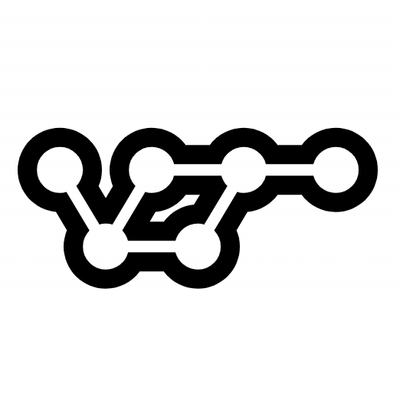
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Shieldify is a Ruby on Rails user authentication plugin. It handles authentication through email and API authentication via JWT (JSON Web Tokens). The gem includes functionalities for user registration, email confirmation, and user authentication via email/password and JWT.
Authentication:
User Management:
To install the Shieldify gem, follow these steps:
Add the gem to your Gemfile:
gem 'shieldify'
Run bundle install
to install the gem:
bundle install
Run the Shieldify install generator to set up the necessary files and configurations:
rails generate shieldify:install
The rails generate shieldify:install
command performs the following steps:
Copy Initializer:
config/initializers/shieldify.rb
Generate Migration:
db/migrate/[timestamp]_shieldify_create_users.rb
email
, password_digest
, email_confirmation_token
, email_confirmation_token_generated_at
, etc., which are essential for managing user authentication.Generate Model:
app/models/user.rb
Inject Method:
shieldify
method call into the User model to include the necessary Shieldify modules.shieldify email_authenticatable: %i[registerable confirmable]
Copy Mailer Layouts:
app/views/layouts/shieldify
Copy Mailer Views:
app/views/shieldify/mailer
Copy Locale File:
config/locales/en.shieldify.yml
Run the migrations to update your database schema:
rails db:migrate
Configure your mailer settings to ensure that confirmation emails are sent correctly. Update your environment configuration (e.g., config/environments/development.rb
) with the appropriate settings.
config.action_mailer.delivery_method = :smtp
config.action_mailer.smtp_settings = {
address: 'smtp.example.com',
port: 587,
user_name: 'your_email@example.com',
password: 'your_password',
authentication: 'plain',
enable_starttls_auto: true
}
That's it! You have successfully installed and configured the Shieldify gem in your Rails application.
Authentication using email and password in this plugin provides a robust and secure way to verify users and generate JSON Web Tokens (JWT) for session management. This method leverages middleware and Warden strategies to seamlessly handle authentication requests. Below is a detailed overview of how this process works:
Middleware and Warden Strategy:
Authentication is processed through middleware that intercepts requests. If the request ends with /shfy/login
, the Warden strategy is used to authenticate the user. If authentication is successful, a JWT is generated and sent in the response headers as Authorization: Bearer <token>
.
Shieldify Middleware: The middleware intercepts requests to handle authentication based on the URL.
Warden Email Strategy:
The Warden strategy validates the user's credentials (email
and password
) that have to come with the request and, if correct, generates a JWT and sends it in the response headers.
Custom Response: In the controller (not generated by the plugin), you can return whatever you want in the body, customizing the response according to your needs.
render json: { message: 'Authenticated successfully', user: current_user }
# config/routes.rb
post '/shfy/login', to: 'sessions#create'
# app/controllers/sessions_controller.rb
class SessionsController < ApplicationController
def create
# Authentication will be handled by Warden
# Custom Response
render json: { message: 'Authenticated successfully', user: current_user }
end
end
curl -X POST http://localhost:3000/shfy/login -d '{
"email": "user@example.com",
"password": "password"
}' -H "Content-Type: application/json"
With this setup, you can authenticate users using email and password, generate a JWT, and handle the response logic in your sessions controller or any controller.
Authentication using JSON Web Tokens (JWT) in this plugin ensures secure and stateless user sessions. This method leverages middleware and Warden strategies to handle authentication requests seamlessly. Below is a detailed overview of how this process works:
Middleware and Warden Strategy:
Authentication is processed through middleware that intercepts requests containing a JWT in the Authorization
header. If a valid JWT is present, the Warden strategy is used to authenticate the user.
Shieldify Middleware:
The middleware intercepts requests to handle authentication based on the presence of the Authorization
header.
Warden JWT Strategy:
The Warden strategy extracts the JWT from the Authorization
header, validates it, and authenticates the user if the token is valid.
Protected Request:
You can protect specific actions in your controllers by using a before_action
filter that ensures the user is authenticated.
before_action :authenticate_user!
# app/controllers/protected_controller.rb
class ProtectedController < ApplicationController
before_action :authenticate_user!
def index
render json: { message: 'You have accessed a protected resource' }
end
end
curl -X GET http://localhost:3000/protected_resource -H "Authorization: Bearer <your_jwt_token>"
With this setup, you can authenticate requests using JWTs, ensuring secure and stateless authentication in your Rails application.
Tu documentación se ve excelente. Aquí está con algunas pequeñas correcciones para mejorar la claridad y el formato:
The Shieldify::Controllers::Helpers
module, which is already included in ActionController::API
, provides several helper methods to manage user authentication within your controllers. Below is a detailed explanation of each method and how to use them:
current_user
This method returns the currently authenticated user based on the Warden strategy.
Usage Example:
# app/controllers/user_controller.rb
class UserController < ApplicationController
def index
user = current_user
render json: { user: user }
end
end
user_signed_in?
This method checks if a user is currently signed in. It returns true
if a user is signed in, otherwise false
.
Usage Example:
# app/controllers/home_controller.rb
class HomeController < ApplicationController
def index
if user_signed_in?
render json: { message: 'User is signed in' }
else
render json: { message: 'User is not signed in' }
end
end
end
authenticate_user!
This method ensures that a user is authenticated before accessing certain actions. If the user is not signed in, it responds with an unauthorized status.
Usage Example:
# app/controllers/protected_controller.rb
class ProtectedController < ApplicationController
before_action :authenticate_user!
def index
render json: { message: 'You have accessed a protected resource' }
end
end
With these helper methods, you can easily manage user authentication and access control in your Rails application.
The Shieldify::Models::EmailAuthenticatable
module provides email and password authentication functionality for users. It includes methods to authenticate users by their email and password, and uses has_secure_password
for secure password handling.
Email and Password Authentication:
password_digest
attribute.Usage Example:
# app/models/user.rb
class User < ApplicationRecord
include Shieldify::Models::EmailAuthenticatable
# or
shieldify email_authenticatable: %i[]
end
Authenticate by Email:
# Authenticate a user by email and password
user = User.authenticate_by_email(email: 'user@example.com', password: 'password')
if user.errors.any?
# Handle authentication failure
else
# Handle authentication success
end
The Shieldify::Models::EmailAuthenticatable::Confirmable
module implements email confirmation using tokens. It generates confirmation tokens when registering or changing a user's email address, enabling confirmation through a secure process. It includes functionality to automatically send confirmation instructions and manage email confirmations.
Email Confirmation:
Usage Example:
# app/models/user.rb
class User < ApplicationRecord
include Shieldify::Models::EmailAuthenticatable::Confirmable
# or
shieldify email_authenticatable: %i[confirmable]
end
Confirm Email by Token:
# Confirm a user's email by token
user = User.confirm_email_by_token('confirmation_token')
if user.errors.any?
# Handle confirmation failure
else
# Handle confirmation success
end
The Shieldify::Models::EmailAuthenticatable::Registerable
module provides registration functionality for users, including email normalization, validation of email and password, and methods for registering a user and updating their email and password.
User Registration:
Usage Example:
# app/models/user.rb
class User < ApplicationRecord
include Shieldify::Models::EmailAuthenticatable::Registerable
# or
shieldify email_authenticatable: %i[registerable]
end
Register a New User:
# Register a new user
user = User.register(email: 'newuser@example.com', password: 'password', password_confirmation: 'password')
if user.errors.any?
# Handle registration failure
else
# Handle registration success
end
Update Password:
# Update user's password
user.update_password(current_password: 'current_password', new_password: 'new_password', password_confirmation: 'new_password')
if user.errors.any?
# Handle password update failure
else
# Handle password update success
end
Update Email:
# Update user's email
user.update_email(current_password: 'current_password', new_email: 'newemail@example.com')
if user.errors.any?
# Handle email update failure
else
# Handle email update success
end
With these modules, you can easily manage user authentication, email confirmation, and registration within your Rails application.
FAQs
Unknown package
We found that shieldify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.